When creating math animations with Manim, understanding how the Python lambda functions work can be extremely useful.
For example, they can be used to graph and animate various functions on the axis.
When I first came across Python lambda functions I had trouble understanding how they work. But then, as I wrote more and more code to create Manim animations I developed my own, simple way of thinking about them.
So here’s everything you need to know about lambda functions in Python to help you create stunning animations with Manim.
What is a lambda function in Python?
A Python lambda function is a small function that doesn’t have a name and can output one expression.
In Python, we use the word def to define a normal function; for lambda functions, that keyword is lambda.
Understanding Python lambda functions
It’s best to start with a few examples.
Example 1:
ooga_booga = lambda x: x + 10
result = ooga_booga(3)
print(result)
Output: 13
lambda is like saying, “Hey Python, get ready to perform a tiny action for me, get ready to use a simple function that doesn’t even have a name on the spot!”
x is a variable that the function will use.
x + 10 is what the function will do with the variable x. In simple terms, it will take a number, add 10 to it, and give you the result.
In this example, I assigned the lambda function to a variable that I decided to name “ooga_booga”. This allows me to use the lambda function later by calling that variable.
Example 2:
Here I wanted a simple function to check if a number is odd or even.
is_even = lambda x: x % 2 == 0
result = is_even(420)
print(result)
Output: True
lambda calls the lambda function. In this example, I assigned the lambda function to a new variable that I named “is_even”. This is useful because now, whenever I want to use that lambda function I can use my variable to do so.
x is the number we want to check.
x % 2 == 0 is the task the lambda function does. It checks if the remainder of dividing x by 2 equals 0. If it does, then it returns True meaning that the number is even; otherwise, it returns False meaning that it’s odd.
Example 3:
I mentioned before that lambda functions return only one expression but that doesn’t mean that they can’t take in multiple expressions. For example, two numbers.
calculation = lambda x, y: x**y if (x < 5 and y < 5) else x * y
result = calculation(2, 3)
print(result)
Output: 8
Once again, saying lambda calls the lambda function. In this example, I assigned that function to a new variable I decided to name “calculation”.
x and y are the two numbers we want to work with. I will be providing them to the lambda function.
x**y if (x < 5 and y < 5) else x * y is the task the lambda function does once it receives two numbers. It checks if both x and y are less than 5. If they are, it raises x to the power of y (x**y). If not, it multiplies them (x * y).
In this example, I gave the function numbers 2 and 3. Since both of them are less than five it raised 2 to the power of 3. That’s why the result is equal to 8 (2*2*2).
Understanding Python lambda functions for animating graphs in Manim
Manim is a Python library that lets you create animations using code.
In Manim you can plot functions onto axes by first creating the axes with the Axes() command, then creating and plotting the function with the .plot() command.
Let’s focus on creating the function for now because that’s when we’ll most likely need to use the lambda function.
Let’s try to plot a parabola onto an axis.
Example:
from manim import *
class parabola(Scene):
def construct(self):
a = Axes(x_range = (-4, 4), x_length = 6, y_range = (0, 16), tips = False).add_coordinates()
parabola = a.plot(lambda x: x**2, color = BLUE)
self.play(Write(a))
self.play(Write(parabola))
self.wait(2)
Output:
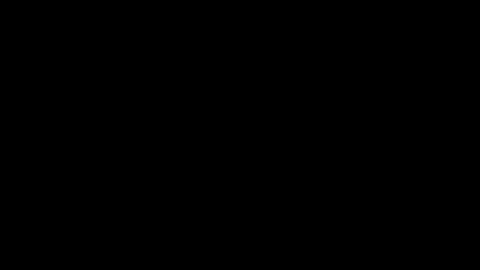
Code explanation:
Line 1: I imported the Manim library into VS Code so that Python understands Manim’s code syntax.
Lines 3 and 4: I created a new Manim scene and named it “parabola”. One scene = one new animation file in Manim.
Line 5: I created axes with x values ranging from -4 to 4 and y values ranging from 0 to 16. I also specified the length of the x-axis to be 6 (which determined how long it is on the screen), removed the arrowhead tips from the end of each axis, and added coordinates so that there are numbers on the axes. Finally, I created a new variable that I called a and assigned my axes to that variable.
Line 7: I plotted a parabola function onto my axis by using the .plot() command. I created the parabola using a lambda function (more on that in a moment). I colored the parabola blue and assigned everything to a new variable that I named parabola.
Line 8: I animated the axis on the screen with self.play() using the Write() animation type.
Line 9: I animated the parabola on the screen using the Write() animation type again.
Line 10: I paused the animation for 2 seconds.
Understanding lambda functions for creating graphs in Manim
So how is this lambda function a parabola:
lambda x: x**2
You can think of it like this:
A traditional parabola function looks like this:
y = x2
or
f(x) = x2
So lambda x: in this case is the same as y or f(x).
In the same way, x**2 is the same as x2. It’s just written in programming terms. Writing it as x*x would mean the same thing.
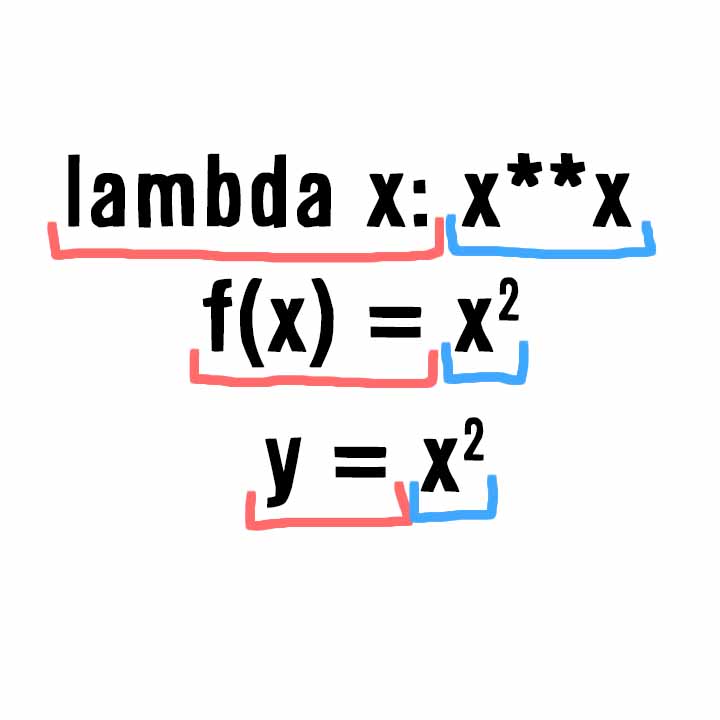
Let me help you learn Manim
If you want to skip the headache of trying to learn Manim from a bunch of scattered information, I put together a comprehensive 3-hour Manim course for complete beginners.
It will give you all the foundational skills you need to start creating stunning animations with code.
Enroll In Manim Course For Beginners