You can plot a function onto axes in Manim by first creating the axes with the Axes() command, and then creating and plotting the function with the .plot() command.
In this guide:
- How to create axes in Manim
- How to plot a parabola function onto axes in Manim
- How to plot a cos function onto axes in Manim
- How to plot a graph in Manim
Let’s look at a few examples:
How to create axes in Manim
To create the axis in Manim use the Axes() command.
Inside the parentheses, you can define a few parameters to customize your axes:
- x_range(x_min, x_max, x_step) – defines the values of the x-axis. Example: x_range(-4, 4)
- y_range(x_min, x_max, x_step) – defines the values of the y-axis. Example: y_range(0, 16)
- x_length – defines the length of the x-axis on the screen. Example: x_length = 6. You can learn more about the screen in Manim here.
- y_length – defines the length of the y-axis on the screen. Example: y_length = 7.
- Tips – lets you enable or disable the tips (arrowheads) at the end of each axis. Example: tips = False.
Example:
from manim import *
class axes(Scene):
def construct(self):
axes = Axes(x_range = (-1, 12), y_range = (-1, 6), tips = False)
axes.add_coordinates()
x_lab = axes.get_x_axis_label("X axis")
y_lab = axes.get_y_axis_label("Y axis")
self.play(Write(axes), Write(x_lab), Write(y_lab))
rec = Rectangle(height = 0.5, width = 0.5)
rec.move_to(axes.c2p(4, 2))
self.play(Write(rec))
self.wait(2)
Output:
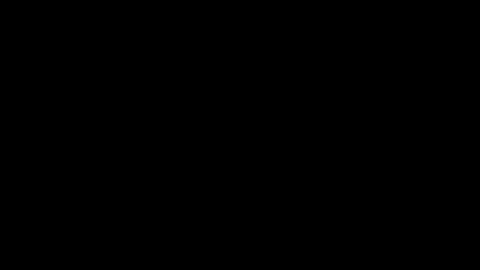
Code explanation:
Line 1: I imported the Manim library into VS Code so that Python understands Manim’s code syntax.
Lines 3 and 4: I created a new Manim scene and named it “axes”.
Line 5: I created the axes with x values ranging from -1 to 12 and y values from -1 to 6. I also disabled the tips on the axes so that the end of each axis doesn’t have an arrow tip and assigned the axes to a new variable that I named “axes”.
Line 6: I added coordinates onto the axes. This added the numbers at each point on each of the x and y axes.
Line 7: I created a label for the x-axis and assigned it to a new variable that I named x_lab.
Line 8: I created a label for the y-axis and assigned it to a new variable that I named y_lab.
Line 10: I animated the axes and the axes labels on the screen using the Write() animation type for each of them.
Line 12: I created a rectangle with a height and width of 0.5. I then assigned it to a new variable that I named rec.
Line 13: I moved the rectangle to coordinates (4, 2) on the axes using the move_to() command. “c2p” stands for “coordinate to point”. Anytime you want to position something on the axes you’ll have to do it like this:
axes_name.c2p(x, y)
Line 15: I animated the rectangle on the screen using the Write() animation type.
Line 16: I paused the animation for 2 seconds.
But in this example, we only created the axes and put a random object in them. Let’s now plot a function onto the axes.
How to plot a parabola function onto axes in Manim
To plot a parabola function onto axes first create the axes with the Axes() command, plot the parabola function onto those axes with the .plot() command, and show everything on the screen with the self.play() command.
Example:
from manim import *
class parabola(Scene):
def construct(self):
a = Axes(x_range = (-4, 4), x_length = 6, y_range = (0, 16), tips = False).add_coordinates()
parabola = a.plot(lambda x: x**2, color = BLUE)
self.play(Write(a))
self.play(Write(parabola))
self.wait(2)
Output:
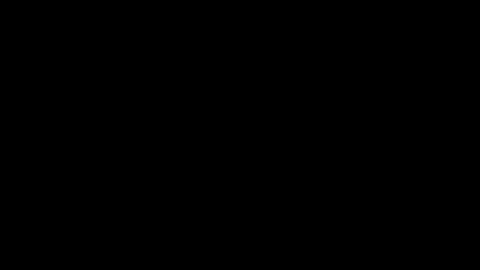
Code explanation:
Line 1: I imported the Manim library into VS Code.
Lines 3 and 4: I created a new Manim scene and named it “parabola”.
Line 5: I created an axis with x values ranging from -4 to 4 and y values ranging from 0 to 16. I specified the length of the x-axis to be 6 (which determined how long it is on the screen), then removed the arrowhead tips from the end of each axis, and added coordinates so that there are numbers on the axes. Finally, I created a new variable that I called a and assigned my axes to that variable.
Line 7: I plotted a parabola function onto my axis by using the .plot() command. I created the parabola using a lambda function. I colored the parabola blue and assigned everything to a new variable that I named parabola.
You can think of this lambda function like this:
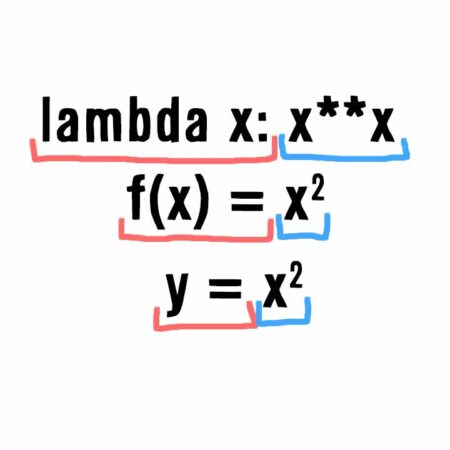
A traditional parabola function looks like this:
y = x2
or
f(x) = x2
So lambda x: in this case is the same as y or f(x).
In the same way, x**2 is the same as x2. It’s just written in programming terms. Writing it as x*x would mean the same thing.
Line 8: I animated the axis on the screen with self.play() using the Write() animation type.
Line 9: I animated the parabola on the screen using the Write() animation type again.
Line 10: I paused the animation for 2 seconds.
How to plot a cosine function onto axes in Manim
To plot a cos function onto axes in Manim you’re going to need to import the numpy library as well as the Manim library. Then, you’ll need to create the axes with the Axes() command, plot the cosine function onto those axes with the .plot() command, and show everything on the screen with the self.play() command.
Example:
from manim import *
import numpy as np
class cos(Scene):
def construct(self):
axes = Axes(x_range = (-12, 12), y_range = (-1, 1), y_length = 2 ,tips = False)
axes.add_coordinates(font_size = 24)
self.play(Write(axes))
cos = axes.plot(lambda x: np.cos(x), color = RED)
self.play(Write(cos))
self.wait(2)
Output:
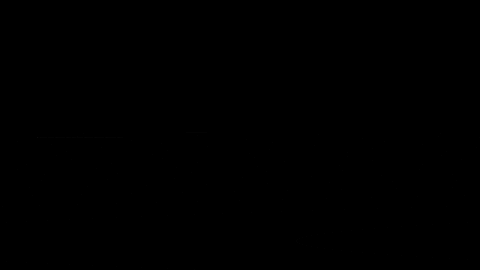
Code explanation:
Line 1: I imported the Manim library into VS Code.
Line 2: I imported the numpy library into VS Code so that I can use the cosine function.
Lines 4 and 5: I created a new Manim scene and named it “cos”.
Line 6: I created the axes onto which I’ll plot my cos function later. I choose the x values to go from -12 to 12, the y values to go from -1 to 1, and the y-axis length to be 2. That way it doesn’t stretch up to the top and the bottom of the screen. I also disabled the tips and assigned my axes to a new variable that I named axes.
Line 7: I added the coordinates onto the axes but I wanted the text to be a little smaller so I also defined the font size to be 24.
Line 9: I animated the axes on the screen using the Write() animation type.
Line 11: I plotted the cosine function onto the axes. I created the cosine function with the help of a lambda function. You can think of the “lambda x:” part as “f(x)” in a traditional function, and “np.cos(x)” as “cos(x)” in a traditional function. So lambda x: np.cos(x) is the same as f(x) = cos(x) or y = cos(x). I then assigned everything to a new variable that I named cos.
Line 13: I animated the cosine function on the screen using the Write() animation type.
Line 14: I paused the animation for 2 seconds.
How to plot a graph in Manim
You can also plot custom graphs in Manim by creating the axes, defining the x and y values, and then plotting them onto those axes.
Example:
from manim import *
class ManimGraph(Scene):
def construct(self):
axes = Axes(x_range = (0, 10), y_range = (10, 70, 10), tips = False)
axes.add_coordinates()
name = Text("Graph").next_to(axes, UP, buff = 0)
x = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
y = [25, 22, 21, 26, 33, 37, 21, 27, 29, 36, 32]
graph = axes.plot_line_graph(x, y, add_vertex_dots=False, line_color = RED)
self.play(Write(axes), Write(name))
self.play(Write(graph))
self.wait(3)
Output:
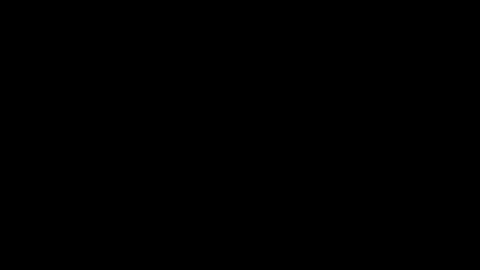
Code explanation:
Line 1: I imported the Manim library into VS Code.
Lines 3 and 4: I created a new Manim scene and named it “ManimGraph”.
Line 5: I created the axes onto which I’ll plot the graph later. I choose the x values to go from 0 to 10, and the y values to go from 10 to 70 in steps of 10 (the third number defines the steps). I also disabled the tips at the end of each axis and assigned everything to a new variable that I named axes.
Line 6: I added the coordinates onto the axes so that the numbers are visible next to each x and y step.
Line 8: I created a new piece of text that said “Graph” and positioned it on top of the axes with the next_to() command and a buff value of 0. To learn more about positioning in Manim I recommend checking out this guide: Manim: How To Align Objects. The buff value defines the gap between two objects, in this case between the axes and the text. I also assigned this text to a new variable that I named name.
Line 10: I created a new variable that I named x and assigned all of my graph’s x values to it.
Line 11: I created a new variable that I named y and assigned all of my graph’s y values to it.
Line 12: I used the plot_line_graph() command to plot the x and y values onto the axes. I also removed the vertex dots (dots at each data point) as well as colored the graph red.
Line 14: I used the self.play() command and the Write() animation type to animate the axes and the name appearing on the screen.
Line 15: I used the Write() animation type to animate the graph appearing on the screen.
Line 16: I pause the animation for 3 seconds.
Let me help you learn Manim
If you want to skip the headache of trying to learn Manim from a bunch of scattered information, I put together a comprehensive 3-hour Manim course for complete beginners.
It will give you all the foundational skills you need to start creating stunning animations with code.
Enroll In Manim Course For Beginners