The difference between the shift and move_to commands in Manim is that shift moves a mobject relative to its current position, and move_to moves a mobject into a specific place in the Manim coordinate system without considering its current position.
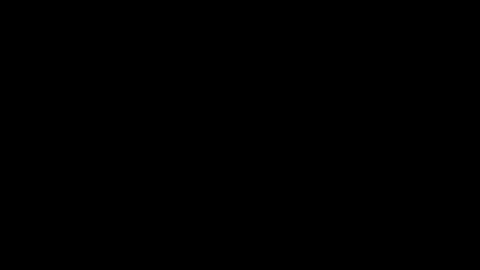
Code:
from manim import *
class move_to_and_shift(Scene):
def construct(self):
d1 = Dot(radius = 0.5, color = RED, stroke_opacity = 1, stroke_color = WHITE, stroke_width = 3)
d2 = Dot(radius = 0.5, color = BLUE, stroke_opacity = 1, stroke_color = WHITE, stroke_width = 3)
t1 = Text("shift", font_size = 69).to_edge(UP)
t2 = Text("move_to", font_size = 69).to_edge(UP)
self.play(Write(d1), Write(t1))
for i in range(3):
self.play(d1.animate.shift(LEFT))
self.wait()
self.play(ReplacementTransform(t1, t2), FadeOut(d1), Write(d2))
for i in range(3):
self.play(d2.animate.move_to(LEFT))
self.wait()
self.play(Unwrite(t2), Unwrite(d2))
Code explanation:
Line 1: I imported the Manim library into VS Code so that Python understands Manim’s code syntax.
Lines 3 and 4: I created a new Manim scene named “move_to_and_shift”.
Line 5: I created a dot and assigned it to a new variable named d1. I then set its radius to 0.5, color to red, stroke opacity to 1 (100%), stroke color to white, and stroke width to 3 so that the outline of the dot becomes visible.
Line 6: I created a second dot and assigned it to another variable d2. I copied all the attributes from the first dot except the color which I set to blue.
Line 8: I created a new piece of text and assigned it to a new variable t1. I said that the text should say “shift” and have a font size of 69. I then moved this text to the top of the screen by using the .to_edge() command.
Line 9: I created another piece of text and assigned it to a new variable t2. I copied all the attributes from the first text, except changing the text itself to say “move_to” instead of “shift”.
Line 11: I used the Write() animation type to animate the first dot (d1) and the first text (t1) on the screen. I separated both animations with a comma and put them inside the same self.play() command so that they appear on the screen at the same time.
Lines 13 and 14: I created a new Python loop that loops what’s inside of it 3 times. Inside of the loop, I said that it should animate the first dot (d1) shifting to the left. The shift will happen 3 times because the loop loops 3 times. Learn more about how to create objects with loops in Manim here.
Line 16: I paused the animation on the screen for 1 second.
Line 18: Inside of the same self.play() command I animated the first piece of text (t1) transforming into the second one (t2), faded out the first dot (d1) using the FadeOut() command, and animated the second dot (d2) on the screen using the Write() animation.
Lines 20 and 21: I created another Python loop that loops what’s inside of it 3 times. This time, inside of the loop, I said it should animate the second dot (d2) moving to “LEFT”. It will move to that location three times because the loop loops three times. But since it’s the move_to() command, the dot will actually stay in place once it moves there. The word “LEFT” in this case specifies the coordinates x = -1; y = 0; z = 0; in the Manim coordinate system.
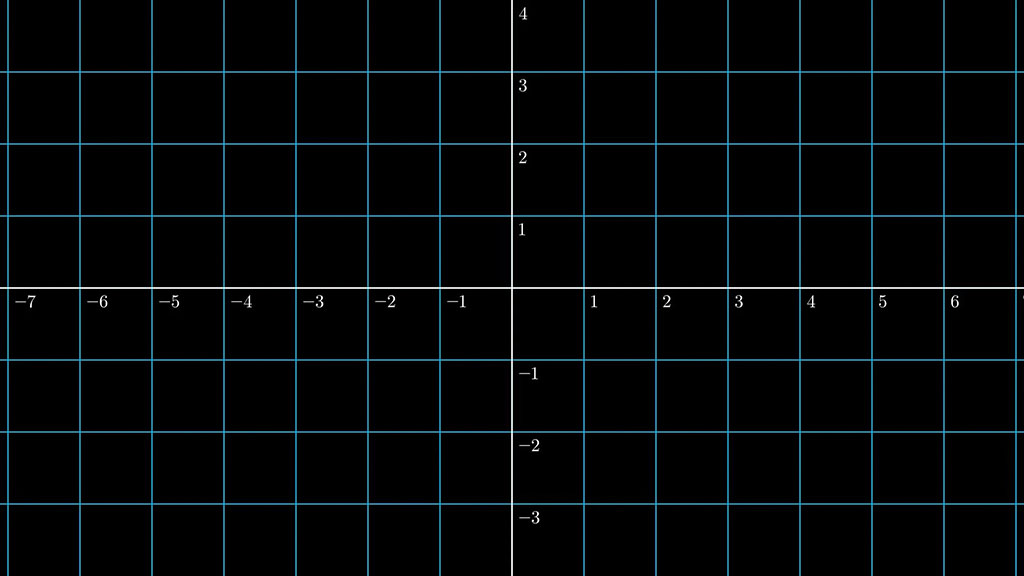
So if I use the .shift(LEFT) command it will shift a mobject left three times, or in other words by three squares from its current position. And if I use the move_to(LEFT) command, it will move it to the position of “LEFT”, which is one square to the left of the center.
Line 23: I paused the animation on the screen for 1 second.
Line 25: I used the Unwrite() animation to remove the second text (t2) and the second dot (d2) from the screen.
Let me help you learn Manim
If you want to skip the headache of trying to learn Manim from a bunch of scattered information, I put together a comprehensive 3-hour Manim course for complete beginners.
It will give you all the foundational skills you need to start creating stunning animations with code.
Enroll In Manim Course For Beginners