In this guide:
What is a VGroup in Manim?
A VGroup in Manim allows you to group multiple objects. It’s useful if you want to move, align, change colors, transform, or in any other way animate multiple objects together on the screen.
You can think of it as a layer group in Photoshop. Every effect you apply to the group applies to all layers inside. The same goes for a VGroup. Everything that you do to a VGroup affects all objects that are inside of it.
How to use a Manim VGroup
How to group objects in Manim
You can group objects in Manim by using a VGroup() command.
Example:
from manim import *
class group(Scene):
def construct(self):
c = Circle(color = RED)
s = Square(color = BLUE).next_to(c, LEFT)
r = RegularPolygon(color = GREEN).next_to(c, RIGHT)
shapes = VGroup(c, s ,r)
self.play(Write(shapes))
self.wait(3)
Output:
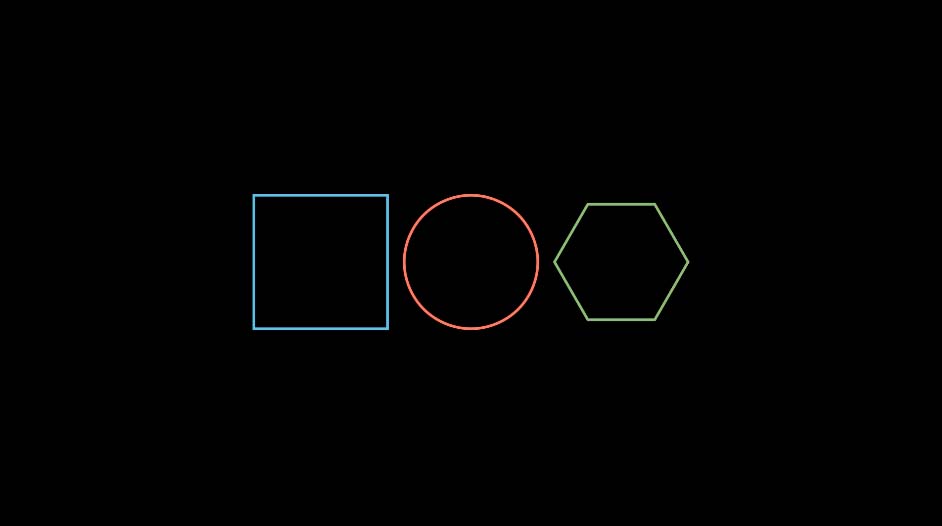
Explanation of each line of code:
Line 1: I imported Manim into my Python file so that VS Code understands Manim code syntax.
Lines 3 and 4: I created a new scene and called it “group”.
Line 5: I created a circle, colored it red, and assigned it to a new variable that I called c.
Line 6: I created a square, colored it blue, and assigned it to a new variable that I called s. I then positioned this square next to the circle on the left side of it by using the .next_to() command. It works like this: .next_to(what, on_which_side).
Line 7: I created a polygon, colored it green, and assigned it to a new variable that I called r. Then I positioned it next to the circle on the right side.
Line 8: I put all of the shapes that I created into a VGroup.
Line 9: I animated the whole group on the screen at the same time by using the Write() animation type.
Line 10: I paused the animation for 10 seconds.
If you think it didn’t make much sense to put all of these objects in a group since I already arranged them before with the .next_to() command, you’re absolutely right.
In this example, grouping all of the shapes allowed me to animate all of them appearing on the screen at the same time.
I’ll show you how to arrange objects in a much more efficient way in a moment.
How to move multiple objects together in a VGroup
You can move multiple objects in Manim by grouping them with VGroup() and then animating the position of that VGroup.
Example:
from manim import *
class move_group(Scene):
def construct(self):
c = Circle(color = RED)
s = Square(color = BLUE).next_to(c, LEFT)
r = RegularPolygon(color = GREEN).next_to(c, RIGHT)
shapes = VGroup(s, c ,r)
self.play(Write(shapes))
self.play(shapes.animate.shift(UP))
self.play(shapes[1].animate.shift(DOWN*1))
self.play(r.animate.shift(DOWN*2))
self.wait(3)
Output:
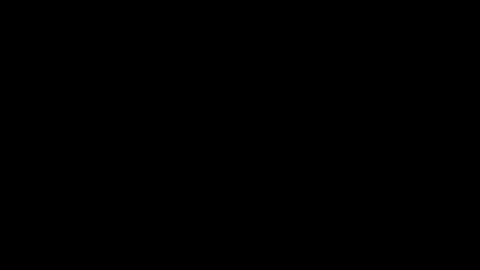
Explanation of each line of code:
Line 1-9: These lines are directly copied from the previous example.
Line 10: I animated the whole group of shapes shifting up using the .shift() command. Since I wanted to show the movement happening on the screen I put everything inside of the self.play() command.
Line 11: Elements in a Manim group act just like arrays in Python so you can animate each object individually even if they’re in a group. In this case, I animated the first element which was the circle (the first element is the circle because the first object in the array always has the index 0). I animated it shifting down using the .shift() command. I also put it inside of the self.play() command because I wanted to show the movement happening on the screen.
Line 12: I animated the polygon shifting down twice the distance of the circle using the .shift() command.
Line 13: I paused the animation for 3 seconds.
With this example, I wanted to show the different ways you can move objects in a VGroup separately.
The first way is to select the object you want to move from the VGroup the same way you would select an element in a regular Python array.
And the second way is to just move that object ignoring that it’s inside of a VGroup. It will stay in that VGruop even if you change its position.
How to align and arrange objects in a VGroup
You can align multiple objects in a VGroup by using the .arrange() command and defining how you want to align them.
I recommend checking out this guide about how to align objects in Manim to learn more in-depth about positioning objects next to each other as well as aligning them.
Example 1:
from manim import *
class text(Scene):
def construct(self):
t1 = Text("Somebody once told me")
t2 = Text("The world is gonna roll me")
t3 = Text("I ain't the sharpest tool in the shed")
g = VGroup(t1, t2, t3)
g.arrange(DOWN, aligned_edge = LEFT)
self.play(Write(g))
self.wait(3)
Output:
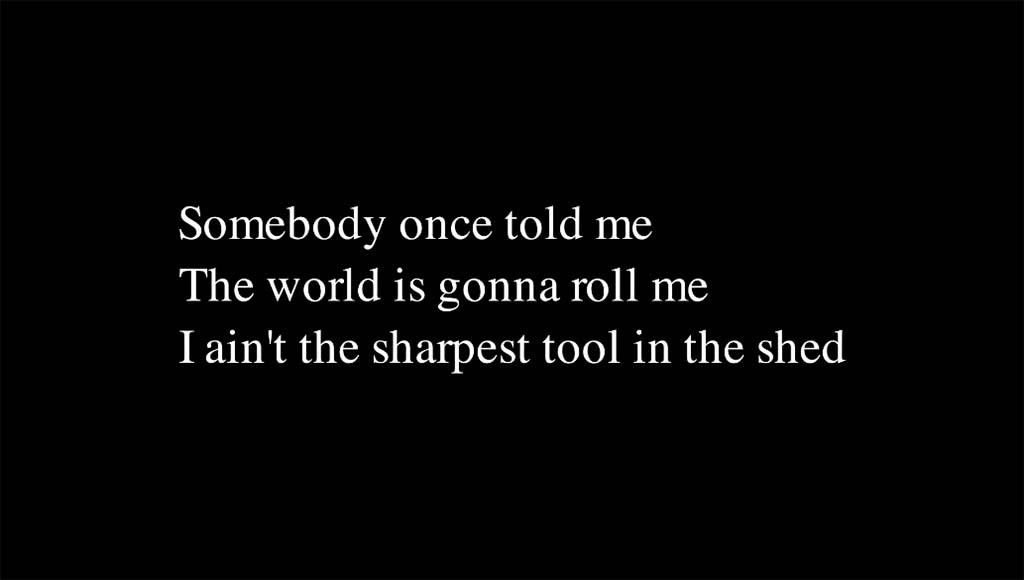
Code explanation:
Line 1: I imported the Manim library into Python.
Lines 3 and 4: I created a new scene and called it “text”.
Lines 5, 6, and 7: I created three new variables (t1, t2, and t3) and assigned a different piece of Text() to each one.
Line 8: I put all three text variables inside of a VGroup and assigned the VGroup to a new variable that I decided to name g.
Line 9: I arranged all the objects inside of the VGruop. I chose to arrange them down and align them to the left edge. If you leave the parentheses empty, it will arrange everything left to right and put the center of all objects in the center of the frame.
Line 10: I animated the VGruop with my text on the screen using the Write() animation type.
Line 11: I paused the animation for 3 seconds.
Example 2:
from manim import *
class aligning(Scene):
def construct(self):
d1 = Dot(color = RED)
d2 = Dot(color = BLUE)
s1 = SurroundingRectangle(d1,
corner_radius = 0.1,
color = RED,
buff = 0.15)
s2 = SurroundingRectangle(d2,
corner_radius = 0.1,
color = BLUE,
buff = 0.15)
t1 = Text("Yikes")
t2 = Text("Sike")
g1 = VGroup(d1, s1)
g2 = VGroup(d2, s2)
g3 = VGroup(g1, t1)
g4 = VGroup(g2, t2)
g3.arrange()
g4.arrange()
g_all = VGroup(g3, g4)
g_all.arrange(DOWN, aligned_edge = LEFT, buff = 0.5)
self.play(Write(g_all))
self.wait(3)
Output:
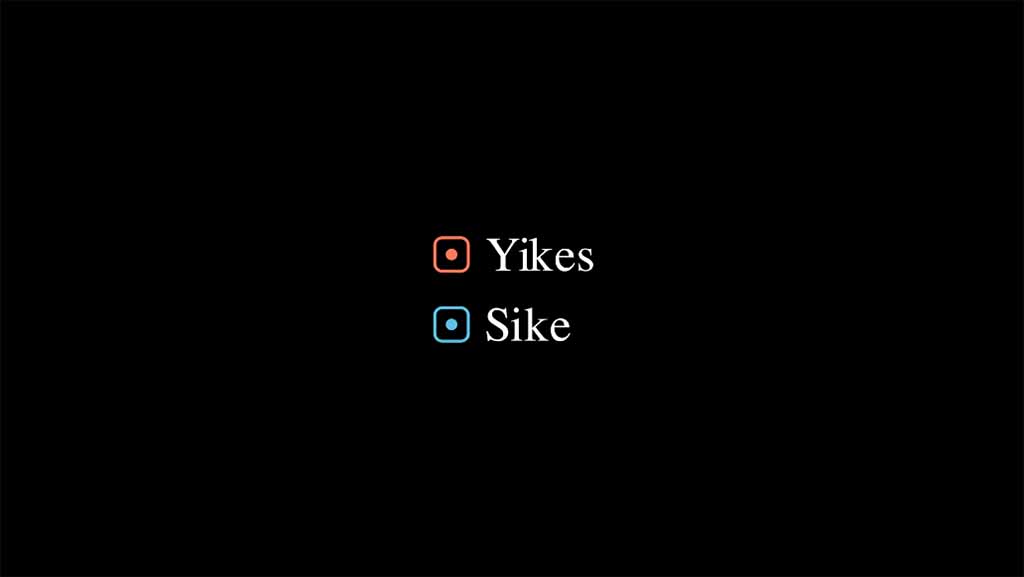
Code explanation:
Line 1: I imported the Manim library into Python.
Lines 3 and 4: I created a new scene and named it “aligning”.
Lines 5 and 6: I created two dots, one red and one blue, and assigned each of them to new variables that I named d1 and d2.
Lines 7 to 14: I created 2 surrounding rectangles and positioned them around each dot. I also said that I want them to have a 0.1 corner radius (this attribute rounds corners), and a 0.15 buff (which defines the distance between the edge of the surrounding rectangle and the object that it surrounds). I also said that I wanted the first surrounding rectangle to be red and the second one to be blue.
Lines 15 and 16: I created two new text variables t1 and t2, and assigned text “Yekes” and “Sike” to each of them.
Lines 18 and 19: Since I wanted to create a “bullet point” from each of the dots and the surrounding rectangles I first needed to put each dot and their surrounding rectangle into a group. So that’s what I did here. The reason for that is that is that I want the dot and the surrounding rectangle to be counted as one object. Later I’ll be aligning each text with their “bullet point” and if I have all of these objects separate then they will align in a straight line.
Lines 20 and 21: Here I grouped each text with their “bullet point”. This is why I grouped the dots with the surrounding rectangles before. I want the dot and the rectangle to count as one object inside of these groups.
Lines 23 and 24: I arranged both of these groups. So now each “bullet point” is next to their text.
Line 26: I grouped both of the text VGroups so that I can arrange them down. If I didn’t arrange them both texts and their bullet points would be in the center of the screen overlapping each other.
Line 27: I arranged both text groups down and aligned them to the left edge.
Line 29: Since I had everything in one group I animated it on the screen using the Write() command. Of course, I could have animated each object individually. That would have made it nicer because I could have used a different animation such as SpinInFromNothing() for the surrounding rectangles.
Line 30: I paused the animation on the screen for 3 seconds.
How to scale objects in a VGroup
You can scale multiple objects in Manim by putting them in a VGroup() and then scaling that VGruop which will scale all the objects that are inside of it.
Example:
from manim import *
class moving_dots(Scene):
def construct(self):
d1 = Dot(color=YELLOW)
d2 = Dot(color=ORANGE)
d3 = Dot(color=RED)
dots = VGroup(d1, d2, d3)
dots.arrange()
self.play(Write(dots))
self.play(dots.animate.scale(10))
self.play(dots.animate.arrange(buff = 1))
self.wait(3)
Output:
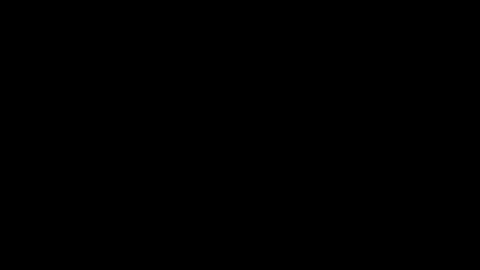
Explanation of each line of code:
Line 1: I imported the Manim library into Python.
Lines 3 and 4: I created a new Manim scene and called it “moving_dots”.
Lines 5, 6, and 7: I created three dots and assigned each of them to a new variable.
Line 8: I grouped all of the dots using a VGruop().
Line 9: I arranged the group with the dots using the default arrange() command. Without any parameters, this command arranges everything left to right and puts the center of all objects in that group in the center of the frame.
Line 10: I used the Write() animation to animate the dots appearing on the screen.
Line 11: I animated the dot group scaling up by 10 times and since I wanted to see the scaling happening on the screen I put everything inside of the self.play() command.
Line 12: I animated the dot group arranging itself on the screen with the buff value of 1. Buff defines the distance between two mobjects.
Line 13: I paused the animation on the screen for 3 seconds.
How to add an object into an existing Manim VGroup
You can add objects to an existing Manim VGroup by using the .add() command.
Example:
from manim import *
class add_objects(Scene):
def construct(self):
d1 = Dot()
d2 = Dot()
d3 = Dot()
g = VGroup(d1, d2, d3)
s = Square()
g.add(s)
g.arrange()
self.play(Write(g))
self.wait(3)
Output:
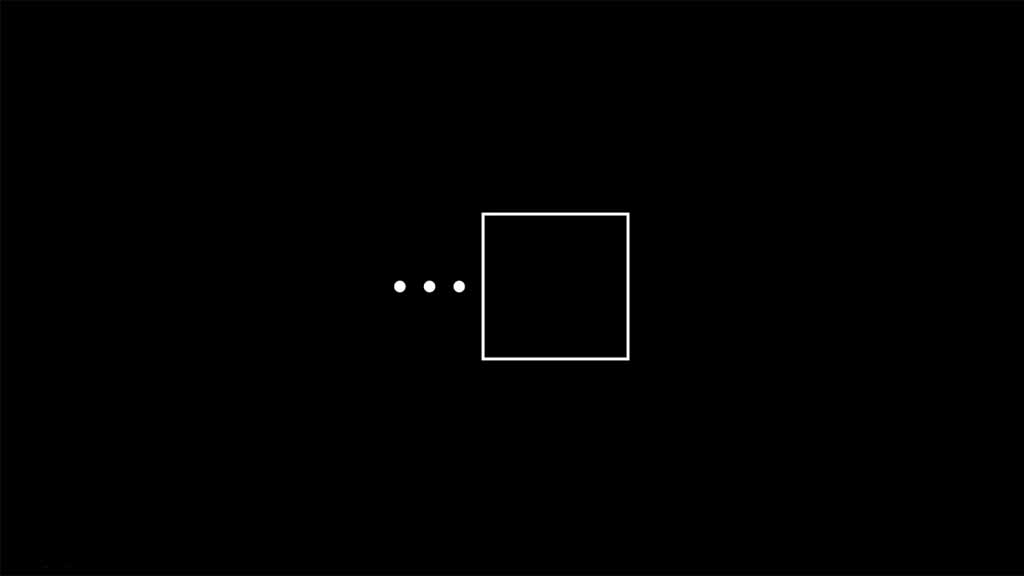
Code explanation:
Line 1: I imported the Manim library into VS Code.
Lines 3 and 4: I created a new Manim scene and named it “add_objects”.
Lines 5, 6, and 7: I created three dots and assigned each of them to a new variable.
Line 8: I put all three dots in a VGroup() and assigned it to a new variable that I named g.
Line 10: I created a square and assigned it to a new variable s.
Line 11: I added the square into the VGruop() with three dots using the .add() command.
Line 12: I arranged the group with all four objects using the default arrangement method which arranges all objects in a VGruop from left to right and centers them in the middle of the frame.
Line 14: I animated the group appearing on the screen using the Write() animation type.
Line 15: I paused the animation for 3 seconds.
Let me help you learn Manim
If you want to skip the headache of trying to learn Manim from a bunch of scattered information, I put together a comprehensive 3-hour Manim course for complete beginners.
It will give you all the foundational skills you need to start creating stunning animations with code.
Enroll In Manim Course For Beginners