The Text object in Manim allows you to animate text.
There are many Text parameters such as font, size, stroke or color as well as many different ways that you can animate your Manim text.
I want to go over the different ways that you can use the Manim Text object for beginners.
Table of contents:
- Manim Text parameters
- How to move Text in Manim
- How to position Manim Text next to another object
- How to change Manim Text
- How to change Manim text alignment
- How to transform Manim text to another object
So let’s start with the parameters.
Manim Text parameters
If you’re using VS Code to write Manim code then every time that you type Text() (or call any other Manim object), Visual Studio Code will show you the parameters that you can use to customize that object.
If you don’t see these parameters you can press Ctrl + Shift + Space or Command + Shift + Space and the parameter hints window will pop up inside of VS Code.
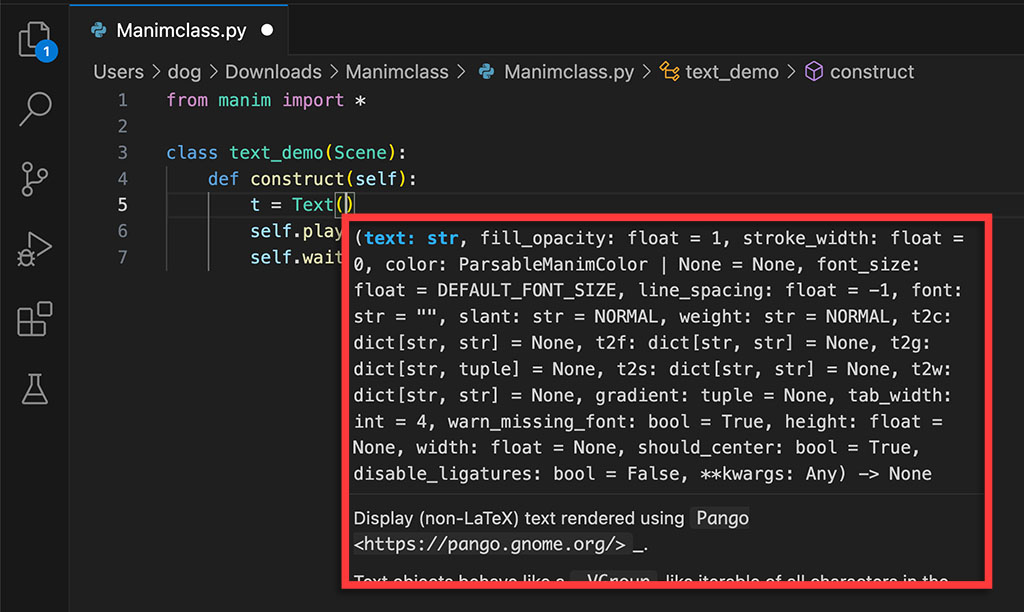
Here are all Manim Text parameters taken from the Manim Community website:
- text (str)
- font (str)
- warn_missing_font (bool)
- fill_opacity (float)
- stroke_width (float)
- color (ParsableManimColor | None)
- font_size (float)
- line_spacing (float)
- slant (str)
- weight (str)
- t2c (dict[str, str])
- t2f (dict[str, str])
- t2g (dict[str, tuple])
- t2s (dict[str, str])
- t2w (dict[str, str])
- gradient (tuple)
- tab_width (int)
- height (float)
- width (float)
- should_center (bool)
- disable_ligatures (bool)
As you can see there are many parameters that you can use for the Manim Text object.
Let’s look at a few examples and how to understand them before we actually use them to animate text.
Example 1:
fill_opacity: float = 1
This means that you can set fill opacity for your Text object and the default value of it is 1.
“Float” means that the value can only be a number or a decimal number (like 0.29, or 3).
You can change this value from 1 to whatever number you want (in this case 1 means 100% so if you pick a number that’s above 1 nothing will visually change, but if you set it to 0.2 for example, the fill opacity will become 20%).
Example 2:
weight: str = NORMAL
This means that you can set the font weight of your text and the default value is “NORMAL”.
“Str” means that the value can only be a string (in other words text).
In this case, if I typed in weight = 3, the program would give me an error.
But how do you find out what kind of other words you can type in?
In this case, since this is font-weight you could probably guess that BOLD would work, but in other cases, it might not be so obvious.
This is where you have to look at the documentation. To do that you can type in the parameter, right-click on it, and choose “Go to definition”.
This will open the documentation of that parameter. Here you can click Ctrl + F or Command + F which will open the search field and look for the documentation of that parameter values.
An alternative way is to Google it.
Example 3:
disable_ligatures: bool = False
“Bool” means that you can assign either “True” or “False” to that parameter (case sensitive).
In this case, the default value is False.
Example 4:
gradient: tuple = None
“Tuple” means that there are multiple items stored in a single variable.
In this case, to color your Manim Text with a gradient you could say:
t = Text("Hello", gradient = (ORANGE, YELLOW))
This would add a gradient to your text from left to right going from color orange to yellow.
Example 5:
t2c: dict[str, str]
“Dict” means that the contents of a dict can be written as a series of key:value pairs within braces { }.
In this case, t2c stands for text to color. You can see that two string values go inside of it indicated by “str”.
This parameter is used to color different words in different colors.
Like so:
from manim import *
class text_demo(Scene):
def construct(self):
t = Text("Hello there", t2c = {"Hello": RED})
self.play(Write(t))
self.wait(3)
Output:
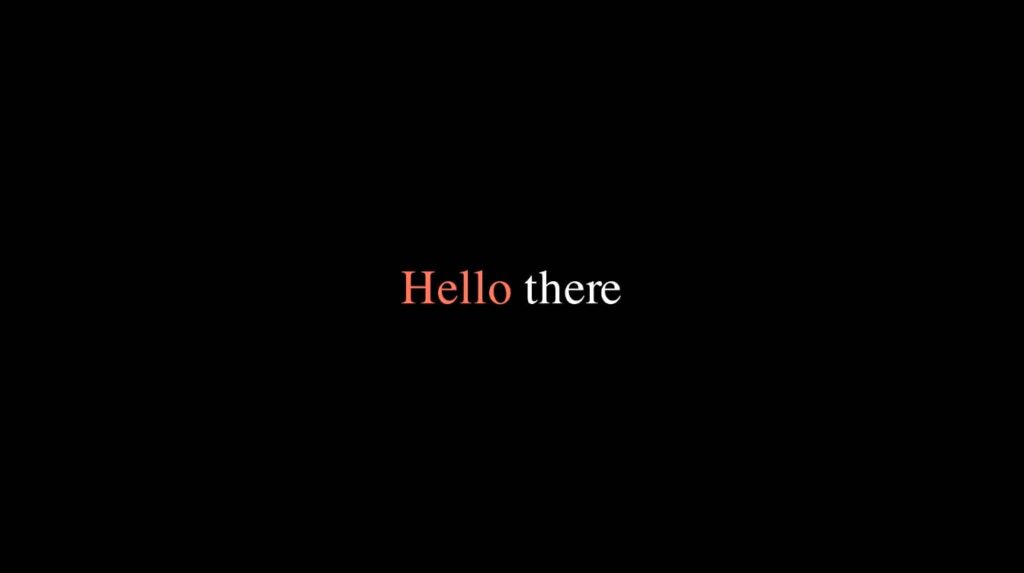
How to move Text in Manim
There are a few ways you can change the position of text inside Manim. Let’s go over each one.
Animate Moving Manim Text with .shift() command
You can use the .shift() function to move your text in any direction you want.
Inside the parentheses, you can input parameters like UP, DOWN, LEFT, and RIGHT. You can also multiply them by a number to indicate how much you want to move your text. By default, it moves by 1.
Example 1:
from manim import *
class text(Scene):
def construct(self):
t = Text("Text").shift(UP)
self.play(Write(t))
self.wait(3)
Output:
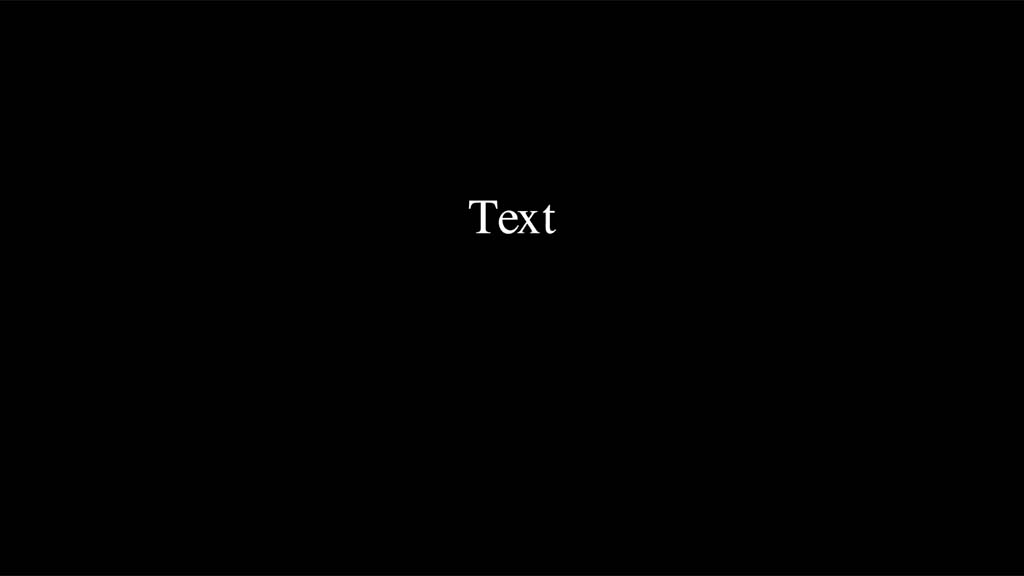
Example 2:
Let’s make text first appear on the screen and then animate moving to another location.
from manim import *
class text(Scene):
def construct(self):
t = Text("Text")
self.play(Write(t))
self.play(t.animate.shift(DOWN*2.5, LEFT*4))
self.wait(3)
In this case, we first created the text, then put it on the screen with self.play(Write(t)) and only then animated it moving from the default position (called ORIGIN) with self.play(t.animate.shift(DOWN2.5, LEFT4)).
When you want to animate text moving, you have to put the command with which you want to move it inside of the self.play() command as well as attach a .animate function to it to animate the movement happening on the screen.
Output:
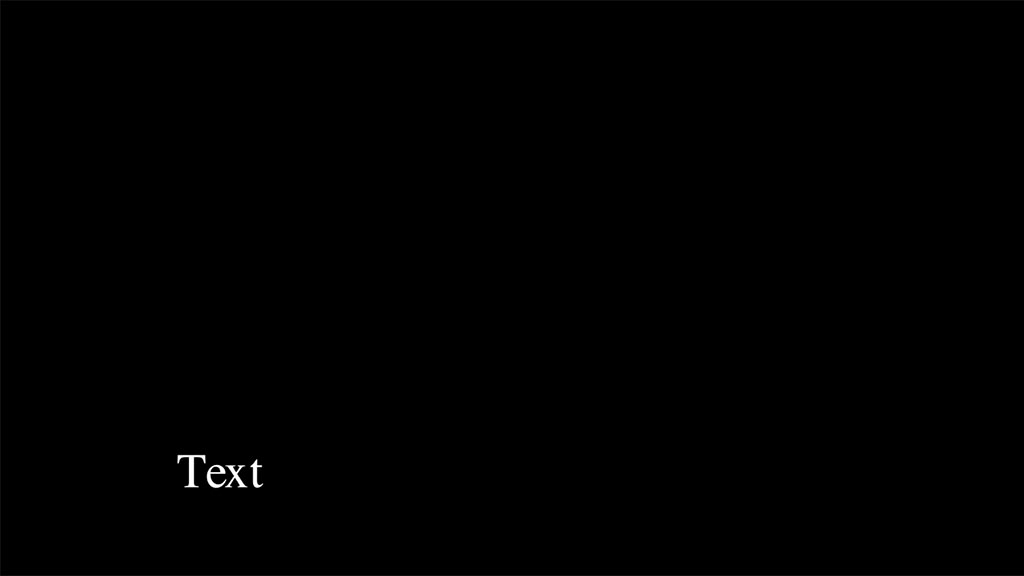
Also, as you can see from the second example you can use multiple parameters inside of the .shift() function to move Manim Text. In this case, the text moved 2.5 squares down and 4 squares left.
Huh? Squares?
By default, the scene in Manim is made up of 8 by 14 squares.
Here’s how the coordinate system (number plane) looks like:
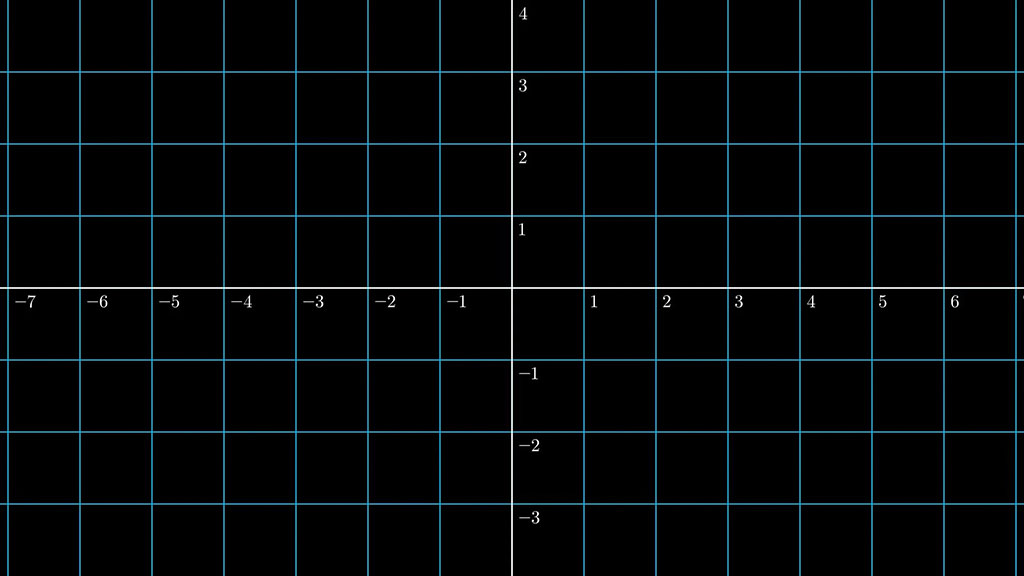
You can show the Mainm coordinate system like this:
from manim import *
class coordinates(Scene):
def construct(self):
c = NumberPlane().add_coordinates()
self.play(Write(c))
So in this case, when we shifted the text down by 2.5 squares and left by 4 squares it ended up here:
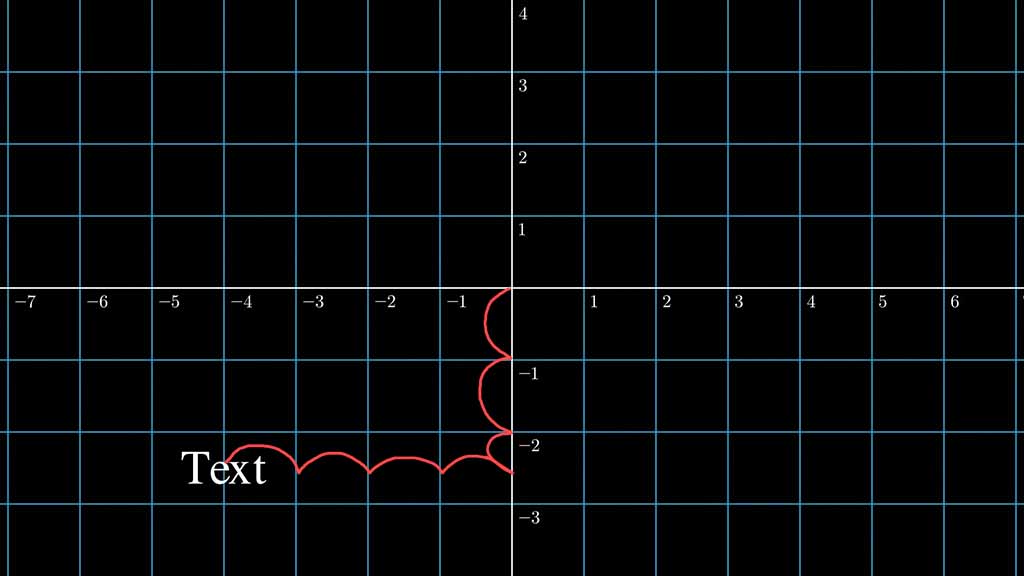
Animate Moving Manim Text with .move_to() command
Now that you’re familiar with the default coordinate system, instead of using the .shift() command you can use the .move_to() command to move text directly to any coordinate you want.
Example:
from manim import *
class text(Scene):
def construct(self):
t = Text("Text")
self.play(Write(t))
self.play(t.animate.move_to([3, -2, 0]))
self.wait(3)
Output:
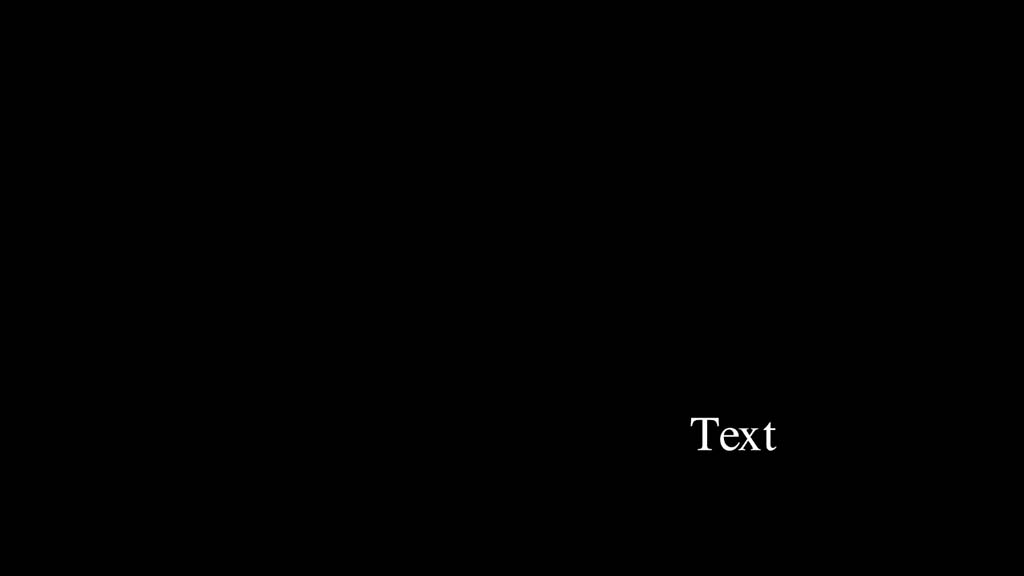
The coordinates look like this:
.move_to([x, y, z])
Z is always 0 since we’re working in a 2D environment.
X moves your Text horizontally.
Y moves your Text vertically.
Also, do not forget to add the square brackets to surround the coordinates.
Once again, in this example, since I put the .move_to() command inside of the self.play() command and attached a .animate command, the text first appeared in the center of the screen and then animated moving to the specified coordinates.
If I wanted the text to appear at those coordinates without moving there from the center I would have written the code like this:
from manim import *
class text(Scene):
def construct(self):
t = Text("Text").move_to([3, -2, 0])
self.play(Write(t))
self.wait(3)
How to position Manim text at the edge of the screen
To position Manim text at the edge of the screen you can use the .to_edge() command.
Inside of the parentheses you have to define which edge you want the text to animate to.
The available parameters are:
- UL – upper left
- TOP – top middle
- UR – upper right
- RIGHT – right side
- DR – down right
- DOWN – down the middle
- DL – down left
- LEFT – left side
Example:
class text(Scene):
def construct(self):
t = Text("Text").to_edge(UR)
self.play(Write(t))
self.wait(3)
Output:
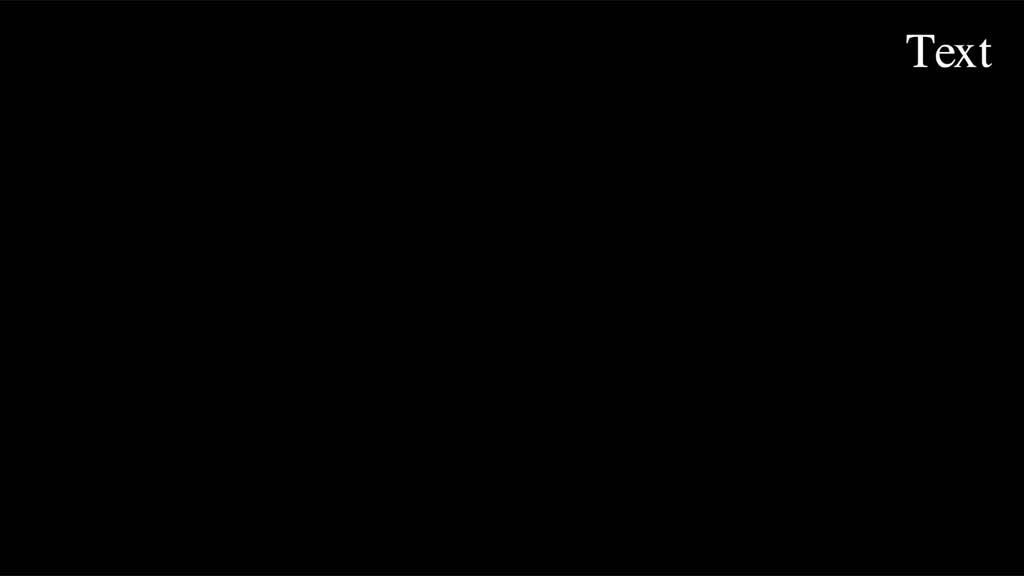
To animate the movement of text you can code it like so:
class text(Scene):
def construct(self):
t = Text("Text")
self.play(Write(t))
self.play(t.animate.to_edge(UR))
self.wait(3)
To learn more about positioning objects in Manim I recommend checking out this guide: Manim: How To Align Objects.
How to Move a part of Manim Text to another place
You don’t have to animate the entire Text moving to another place.
The Text object in Manim is an array.
Which means that you can affect individual letters.
Example:
class text(Scene):
def construct(self):
t = Text("Shrek is love")
self.play(Write(t))
self.play(t[0:5].animate.shift(UP))
self.play(t[7:].animate.shift(DOWN))
self.wait(3)
Output:
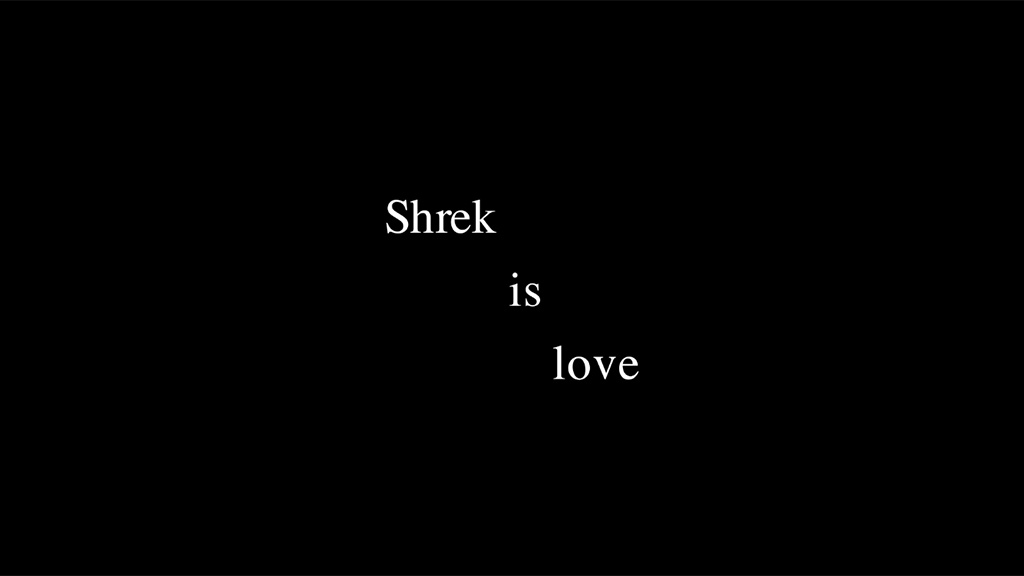
Each item in a Python array has its index. A number that you can call to only affect that item in the array. It starts from 0.
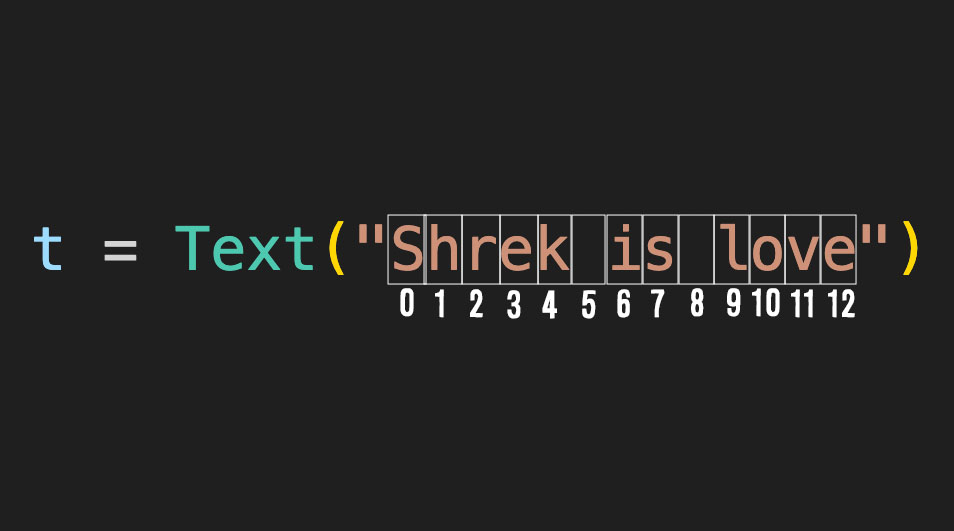
To affect multiple items you can define a range.
In this example, I chose to only move the text “Shrek” by selecting elements 0 to 5 in the Text array.
The last element is NOT INCLUDED.
So by saying t[0:5] I only chose up to the 4th letter which is the letter “k” in the word “Shrek”. The fifth element (the space) was not included in the selection.
class text(Scene):
def construct(self):
t = Text("Shrek is love")
self.play(Write(t))
self.play(t[0:5].animate.shift(UP))
self.play(t[7:].animate.shift(DOWN))
self.wait(3)
You can also affect just one object in the array like this:
t[0].color = RED
This will only color the letter “S” red.
You can affect everything from a certain object onwards like this:
t[5:].color = RED
This will color every element from the 5th one red. So in this example, the words “is love” would be red.
You can affect everything up to a certain object in an array like this:
t[:5].color = RED
This will color everything up to the fifth element in the array. So the word Shrek will be red.
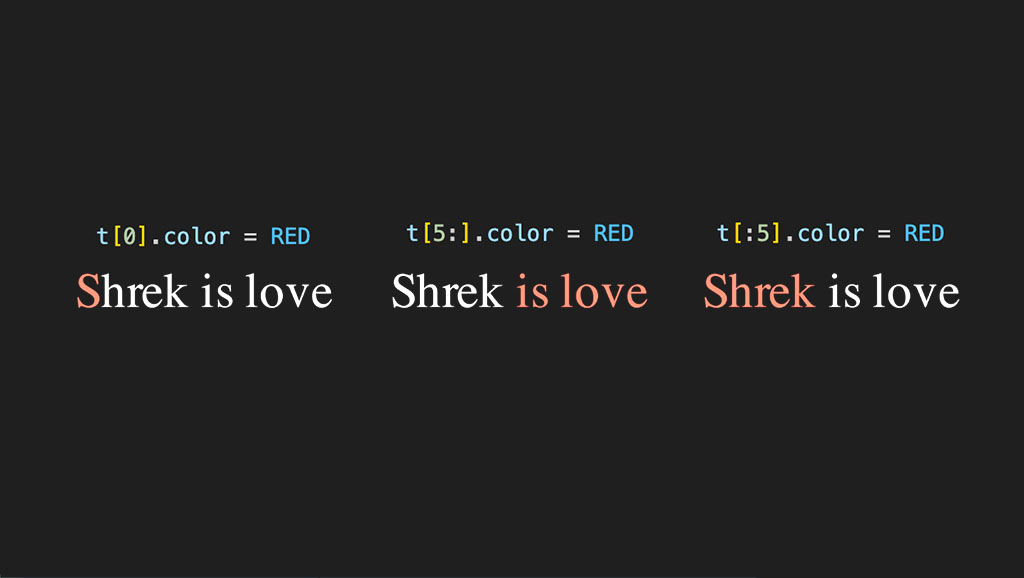
How to position Manim Text next to another object
You can use the .next_to() command to position Manim Text next to another object.
Example:
from manim import *
class text(Scene):
def construct(self):
s = Square(color = RED, fill_opacity = 1).shift(UP, LEFT*3)
t = Text("Hahaha").next_to(s, RIGHT)
self.play(Write(t), Write(s))
self.wait(3)
Output:
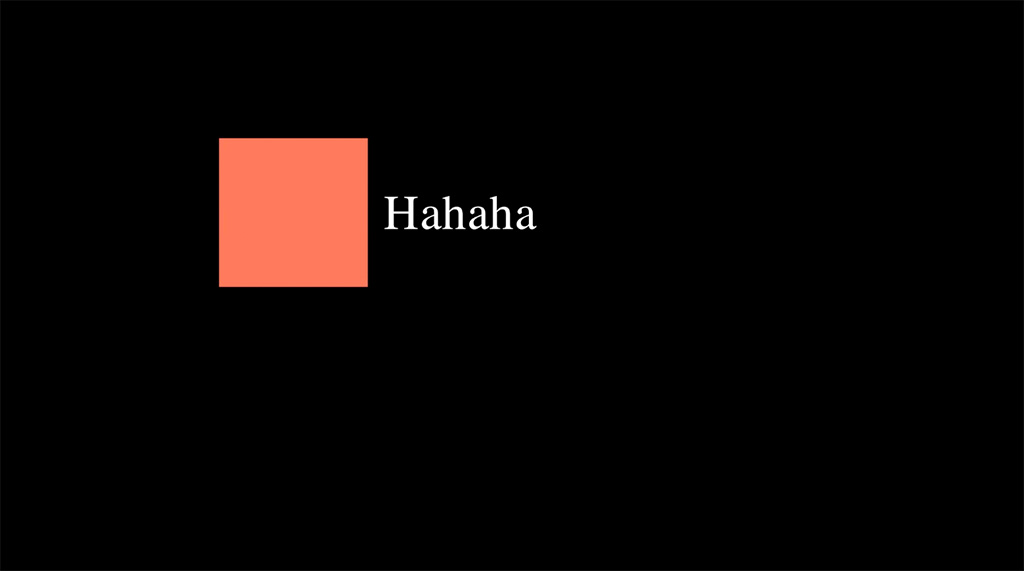
Here’s how it works:
.next_to(which_object, which_side)
How to change Manim Text
To change from one Manim text to another you can use the Transform() command.
Example:
from manim import *
class text(Scene):
def construct(self):
t1 = Text("Hello")
t2 = Text("I'm Shrek")
self.play(Write(t1))
self.play(Transform(t1, t2))
self.wait(3)
In this example first the text “Hello” will appear on the screen and then it will animate transforming from “Hello” to “I’m Shrek”.
How to change Manim text alignment
The best way to arrange text in Manim is to put all of your text variables into a group and then arrange objects in that group.
To learn more about positioning I recommend checking out this guide on how to align objects in Manim.
How to arrange text vertically in Manim
Let’s look at how to align multiple Manim Text objects to the left edge:
First, if you have multiple Text objects you can put them into a group with a VGroup() command and assign that group to another variable.
Then you can arrange all objects inside of that group to the left edge.
Example:
from manim import *
class text(Scene):
def construct(self):
t1 = Text("Hello there")
t2 = Text("I'm Shrek")
t3 = VGroup(t1, t2)
t3.arrange(DOWN, aligned_edge = LEFT)
self.play(Write(t3))
self.wait(3)
Output:
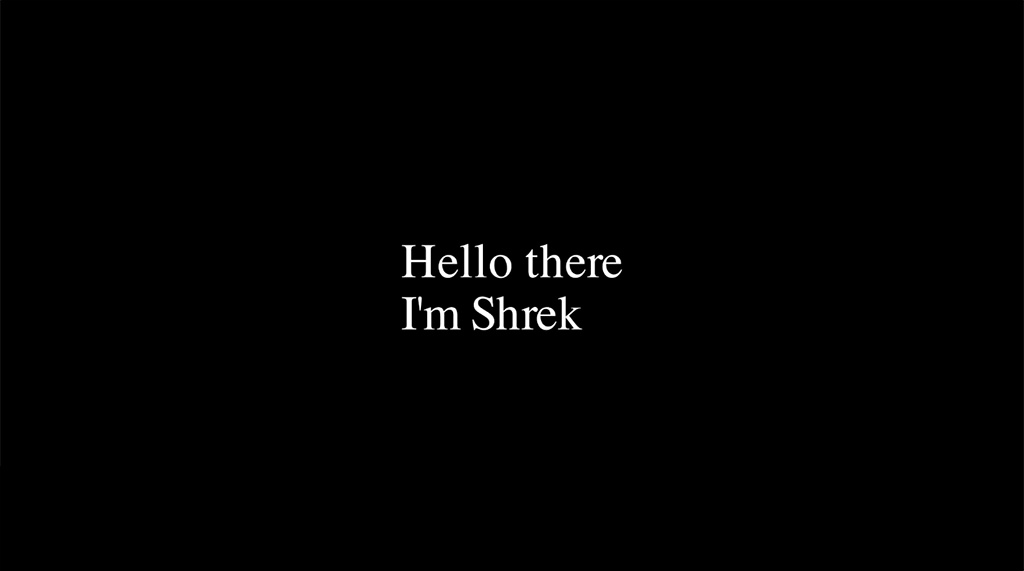
How to arrange text horizontally in Manim
Here’s how to align multiple Manim text objects horizontally so that their center is in the center of the screen:
class text(Scene):
def construct(self):
t1 = Text("Hello there")
t2 = Text("I'm Shrek")
t3 = VGroup(t1, t2)
t3.arrange()
self.play(Write(t3))
self.wait(3)
Output:
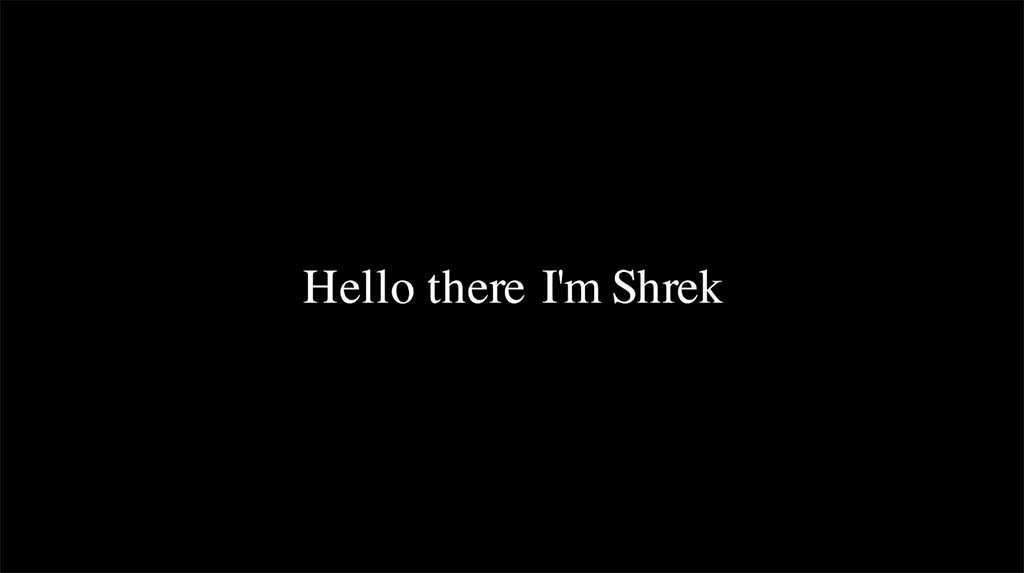
In this case, I didn’t need to put any parameters inside of the .arrange() function because by default it arranged all objects within a VGroup from left to right and puts them in the center of the screen.
How to transform Manim text to another object
To transform text in Manim to another object you can use the Transform() command.
Here’s how it works.
Transform(from_what, to_what).
First, create the object that you want to transform to and then use the Transform() command inside of the self.play() command.
Example:
class text(Scene):
def construct(self):
t = Text("Illuminati")
tri = Triangle(color = GREEN, fill_opacity = 1)
self.play(Write(t))
self.play(Transform(t, tri))
self.wait(3)
In this case, first the text “Illuminati” appears on the screen and then it transforms into a green triangle.
Let me help you learn Manim
If you want to skip the headache of trying to learn Manim from a bunch of scattered information, I put together a comprehensive 3-hour Manim course for complete beginners.
It will give you all the foundational skills you need to start creating stunning animations with code.
Enroll In Manim Course For Beginners