With Manim you can animate complex math equations and formulas using LaTeX. Here’s how.
How to write math formulas in Manim using LaTeX
There are two things to keep in mind before creating any math text with LaTeX:
- To type out math text with Manim you have to use the Tex() command instead of the usual Text() command.
- Before you type math text with LaTeX you need to let Python know to treat the symbols as LaTeX commands and not as Python commands. To do that add a letter r before you type math text in LaTeX.
This creates what’s called an “r-string“. It basically tells Python to treat backslashes (\) as a normal character and not as an escape symbol. - After the letter r add quotation marks and inside of them surround your math text with a dollar symbol $ on each side. The dollar signs distinguish a LaTeX math text from a regular text.
As a side note, you might be getting an error that says “manim latex error converting to dvi“. What worked for me to fix it was to surround the text in dollar signs after the quotation marks: Tex(“$a = b + c$”).
Example:
from manim import *
class Einstein(Scene):
def construct(self):
t = Tex(r"$E=mc^2$", font_size = 96)
self.play(Write(t))
self.wait()
Output:
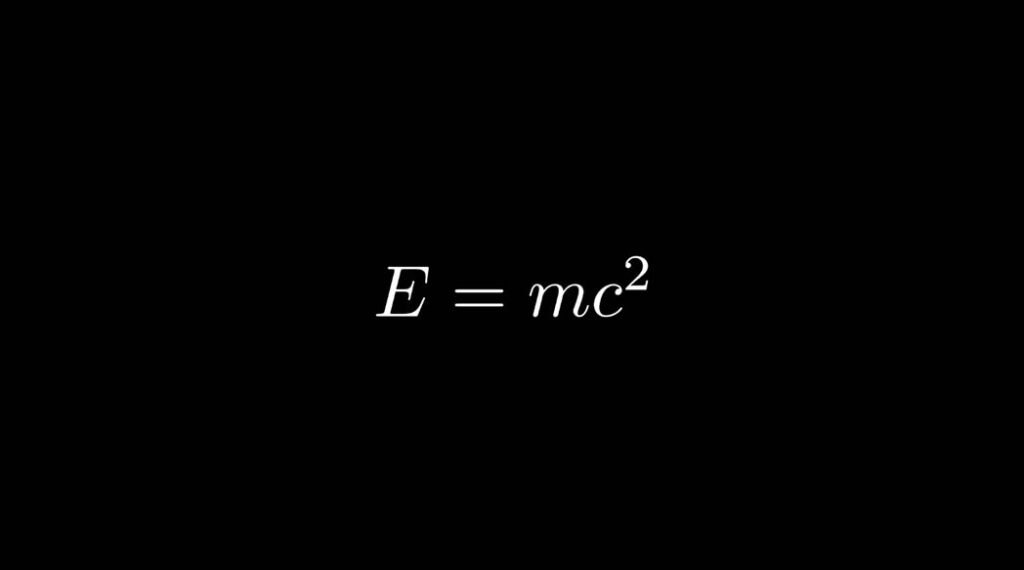
How to write a fraction with LaTeX in Manim
To write a fraction with LaTeX use the \frac{a}{b} syntax. What’s inside the {a} curly brackets will go on top of the fraction (numerator) and what’s inside {b} curly brackets will go at the bottom (denominator).
Example:
from manim import *
class Fraction(Scene):
def construct(self):
t = Tex(r"$(\frac{1}{3})^3 - \frac{5}{3} = -1\frac{17}{27}$", font_size = 96)
self.play(Write(t))
self.wait()
Output:
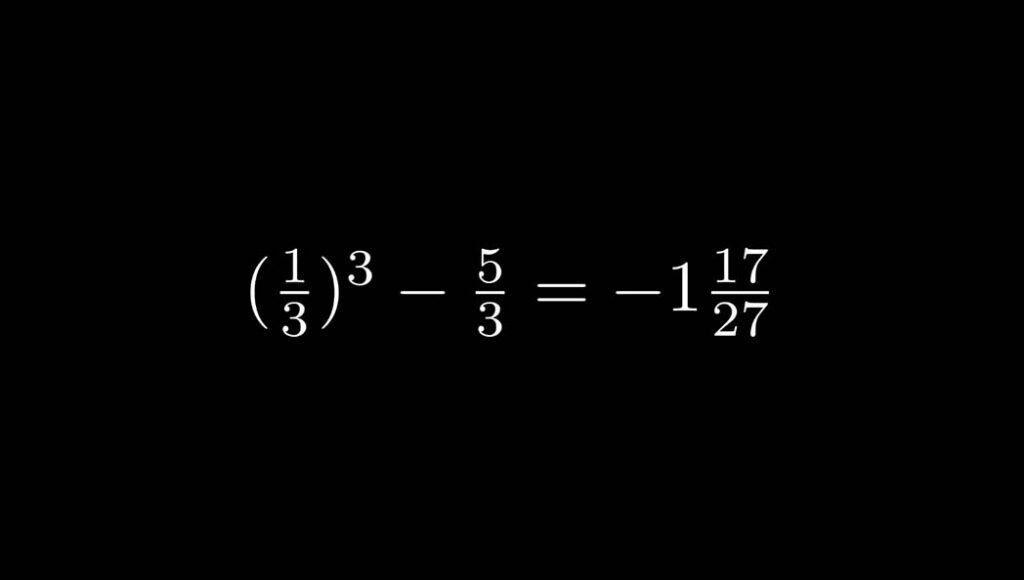
How to animate an equation changing in Manim?
To animate an equation changing in Manim, first create the equation before and after the transformation and assign each of them to a new variable. Then, use the ReplacementTransform, TrasnfromFromCopy, or TransformMatchingTex commands to show the equation changing from the first version to the second one.
from manim import *
class Equation(Scene):
def construct(self):
t1 = Tex(r"$x_{1, 2} =$", r"$\frac{-b \pm \sqrt{D}}{2a}$", font_size = 96)
t2 = Tex(r"$x_{1, 2} =$", r"$\frac{-b \pm \sqrt{b^2 - 4ac}}{2a}$", font_size = 96)
g = VGroup(t1, t2).arrange(DOWN, aligned_edge = LEFT, buff = 1)
self.play(Write(t1))
self.wait(0.5)
self.play(TransformFromCopy(t1[0], t2[0]))
self.play(TransformFromCopy(t1[1], t2[1]))
self.wait()
Output:
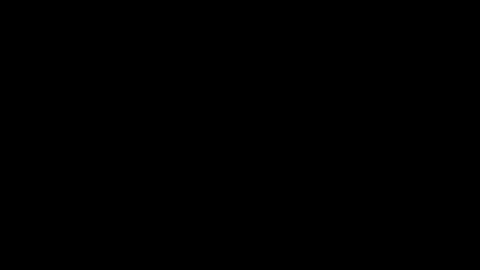
Code explanation:
Line 1: I imported the Manim library into VS Code so that Python understands Manim’s code syntax.
Lines 3 and 4: I created a new Manim scene named “Equation”.
Line 5: I created a new variable t1 and assigned Tex() to it. Tex() is the command you need to use to write LaTeX text with Manim. I put my LaTeX math text inside quotes and surrounded it with dollar signs. Then I said that the font size of it should be 96.
LaTeX explanation:
r"$x_{1, 2} =$", r"$\frac{-b \pm \sqrt{D}}{2a}$"
r – tells Python to treat backslashes (\) as a normal character and not as an escape symbol.
“” – indicates the start and end of a string.
$$ – indicates the start and end of a LaTeX math text.
x_{1, 2} – creates the x with superscript with LaTeX (what’s inside the curly brackets is the tiny text next to the x (superscript)).
\frac{}{} – creates a fraction. What’s on top of the fraction goes into the first curly brackets and what’s at the bottom goes into the second curly brackets.
\pm – creates a +- symbol in LaTeX.
\sqrt{} – creates a square root symbol in LaTeX. What’s inside the square root goes into the curly brackets.
Here is a list of LaTeX math symbols.
Line 6: I created the second equation that I want to transform the first one into. I then assigned it to a new variable t2 and set it to the same font size as the first one.
Line 7: I put both equations into a VGroup() and then arranged that group down and aligned all the objects in it to the left edge. I also passed in a buff value of 1. The buff value defines the space between mobjects.
Line 8: I animated the first equation on the screen using the Write() animation type.
Line 9: I paused the animation on the screen for 0.5 seconds.
Line 10: I used the TransformFromCopy animation to transform the first part of the first equation into the first part of the second equation. When you separate strings with a comma in the Tex() command they become separate elements inside the Tex array. So in this case t1[0] indicated only the part in the equation that was on the left side including the equals sign. The TransformFromCopy command does what it says. It transforms one object into another leaving the first one visible on the screen.
Line 11: I did the same thing for the second part of each equation.
Line 12: I paused the animation on the screen for 12 seconds.
Let me help you learn Manim
If you want to skip the headache of trying to learn Manim from a bunch of scattered information, I put together a comprehensive 3-hour Manim course for complete beginners.
It will give you all the foundational skills you need to start creating stunning animations with code.
Enroll In Manim Course For Beginners