The default scene in Manim is made up of 8 by 14 squares.
This is the coordinate system, also called the NumberPlane() that you can use to position mobjects on the screen.
How to show the Manim coordinate system grid on the screen
You can show Manim coordinates on the screen like this:
from manim import *
class grid(Scene):
def construct(self):
c = NumberPlane().add_coordinates()
self.play(Write(c))
Output:
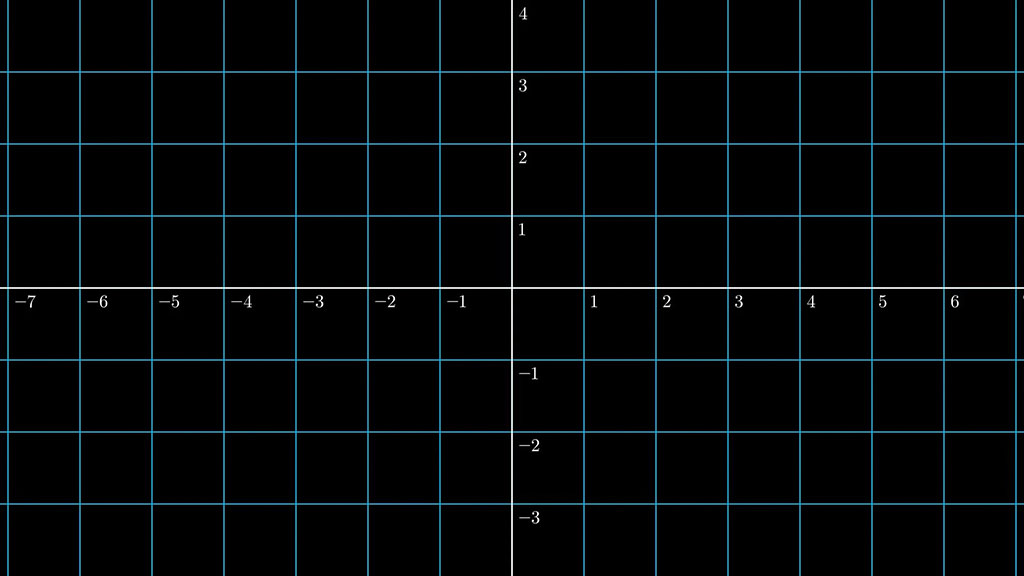
How to move objects to a specific part of the screen in Manim
You can move objects to specific coordinates on the screen using the move_to() command.
Example:
from manim import *
class moving(Scene):
def construct(self):
d = Dot(color = RED, radius = 0.2)
d.move_to([-3, 2, 0])
self.play(Write(d))
self.play(d.animate.move_to([5, -1, 0]))
self.play(d.animate.move_to(ORIGIN))
self.play(Unwrite(d))
Output:
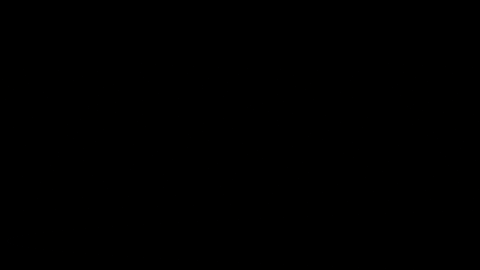
Code explanation:
Line 1: I imported the Manim library into VS Code so that Python understands Manim’s code syntax.
Lines 3 and 4: I created a new Manim scene and named it “moving”.
Line 5: I created a red dot with a radius of 0.2 and assigned it to a new variable that I named d.
Line 6: I used the move_to() command to move that dot to coordinates [x = -3; y = 2; z = 0] (when working with 2D animations the z coordinate will always be 0).
Line 8: I animated the dot on the screen using the Write() animation type.
Line 9: I animated the dot moving on the screen from its previous coordinates to [x = 5; y = -1; z = 0]. When you want to show something happening on the screen you need to put it inside the self.play() command and in the case of moving something also attach the .animate attribute.
Line 10: I animated the dot moving to the ORIGIN which means the center of the screen or the coordinates [0, 0, 0].
Line 11: I animated the dot disappearing using the Unwrite() animation type.
Don’t forget to use the brackets [ ] inside of parentheses ( ) when typing out coordinates inside of the move_to() command. It should look like this:
move_to([x, y, z])
Let me help you learn Manim
If you want to skip the headache of trying to learn Manim from a bunch of scattered information, I put together a comprehensive 3-hour Manim course for complete beginners.
It will give you all the foundational skills you need to start creating stunning animations with code.
Enroll In Manim Course For Beginners