The first way to remove multiple objects from the scene in Manim is to group those objects by using a VGroup() and then remove the entire group, and the second method is to use a loop inside of the self.play() command to loop through every object and fade it out.
How to remove all objects from the screen using a group in Manim
To remove all objects with a group:
- Group the objects that you want to remove with VGroup().
- Inside of the self.play() command use the FadeOut() animation type.
- Specify the name of your group inside of the FadeOut() parentheses.
Example:
from manim import *
class remove_objects(Scene):
def construct(self):
c1 = Circle(0.5, color = RED)
c2 = Circle(0.7, color = ORANGE)
c3 = Circle(0.9, color = YELLOW)
self.play(Write(c1), Write(c2), Write(c3))
self.wait()
g = VGroup(c1, c2, c3)
self.play(FadeOut(g))
Output:
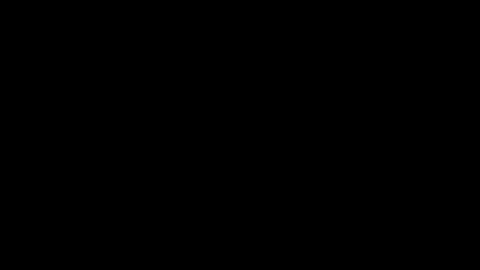
Code explanation:
Line 1: I imported the Manim library into VS Code so that Python understands Manim’s code syntax.
Lines 3 and 4: I created a new Manim scene named “remove_objects”.
Lines 5-7: I created 3 circles (c1, c2, and c3), each with a slightly bigger radius and a different color.
Line 9: I animated all of the circles on the screen at the same time using the Write() animation type. If you want to animate something happening on the screen in Manim you always have to put it inside the self.play() command.
Line 10: I paused the animation for 1 second.
Line 12: I grouped all of the circles using a VGroup() and assigned this group to a new variable that I named g.
Line 13: I removed all of the circles from the screen by fading out the group (that contains all of them) inside of the self.play() command.
How to remove all objects from the screen using a loop in Manim
To remove all objects with a loop use this code at the point in your animation when you want to remove everything from the screen:
self.play(
*[FadeOut(mob)for mob in self.mobjects]
)
This loops through all objects in the scene and once it finds them it then fades them out.
Example:
from manim import *
class remove_objects2(Scene):
def construct(self):
t1 = Triangle(color = TEAL).shift(UP)
t2 = Triangle(color = BLUE).scale(1.2)
t3 = Triangle(color = LIGHT_PINK).scale(1.4).shift(DOWN)
self.play(Write(t1), Write(t2), Write(t3))
self.wait()
self.play(*[FadeOut(mob)for mob in self.mobjects])
Output:
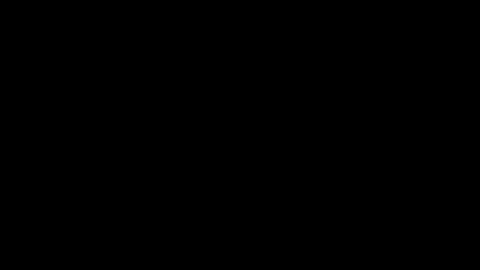
Code explanation:
Line 1: I imported the Manim library into VS Code.
Lines 3 and 4: I created a new Manim scene and named it “remove_objects2”.
Line 5: I created a triangle (t1) and set its color to teal as well as shifted it up on the screen. See Manim coordinate system.
Line 6: I created the second triangle (t2), set its color to blue, and scaled it up by 1.2 times.
Line 7: I created the third triangle (t3), set its color to light pink, scaled it up by 1.4 times, and shifted it down by 1 square in the Manim coordinate system.
Line 9: I animated all of the triangles on the screen at the same time using the Write() animation type for each of them.
Line 10: I paused the animation on the screen for 1 second.
Line 12: I removed all objects on the screen using a loop. This loop found all the objects and removed them using the FadeOut() animation type.
Let me help you learn Manim
If you want to skip the headache of trying to learn Manim from a bunch of scattered information, I put together a comprehensive 3-hour Manim course for complete beginners.
It will give you all the foundational skills you need to start creating stunning animations with code.
Enroll In Manim Course For Beginners