You can highlight objects in Manim by using Circumscribe() or Indicate() commands inside of self.play(). Inside of their parentheses define the object that you want to highlight.
Let’s look at a few examples.
How to highlight objects in Manim
First, let’s learn the basics and highlight entire objects or object groups.
Example:
from manim import *
class highlight(Scene):
def construct(self):
s1 = Square(color = PURPLE)
s2 = Triangle(color = BLUE)
s3 = RegularPolygon(color = TEAL)
g = VGroup(s1, s2, s3)
g.arrange(buff = 1)
self.play(Write(g))
self.play(Indicate(s1))
self.play(Circumscribe(s3))
self.play(Indicate(g, scale_factor = 0.7, color = RED))
self.play(Circumscribe(s2, Circle, stroke_width = 15))
self.wait()
Output:
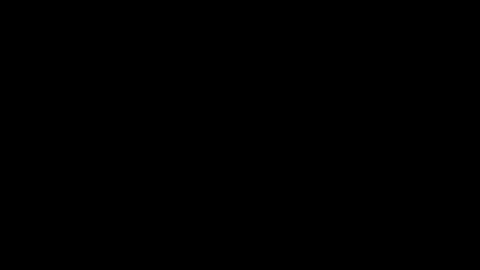
Code explanation:
Line 1: I imported the Manim library into VS Code so that Python understands Manim’s code syntax.
Lines 3 and 4: I created a new Manim scene and named it “highlight”.
Line 5: I created a purple square and assigned it to a new variable s1.
Line 6: I created a blue triangle and assigned it to a new variable s2.
Line 7: I created a polygon and assigned it to a new variable s3.
Line 9: I grouped all of these shapes using a VGroup(). This allows me to arrange all of the objects in that group easier and also animate them on the screen at the same time.
Line 10: I arranged the group by using the default arrangement method which arranges every object from left to right and centers them in the center of the frame. I also added a buff value of 1 which increased the distance between each object.
Line 12: I animated the group of shapes on the screen using the Write() animation type.
Line 13: I highlighted the first shape using the Indicate() command. This command highlights an object by temporarily resizing and coloring it.
Line 14: I highlighted the third shape using the Circumscribe() command. This command highlights an object by drawing a temporary line that surrounds the object.
Line 15: I highlighted the entire group of shapes using the Indicate() command but this time I also defined the scale factor to be less than 1, meaning that it will not expand the object but shrink it down. I also changed the color of the highlight to red instead of the default yellow.
Line 16: I highlighted the second shape using the Circumscribe() command but this time I said that I wanted the shape of the highlight to be a circle instead of a rectangle as well as have a stroke of 15 which made the line thicker.
Line 17: I paused the animation on the screen for 1 second.
How to highlight a part of a text in Manim
Since Text() in Manim acts just like a regular Python array you can highlight a part of a text by defining which objects in a Text() array you want to highlight.
You can learn more about how Manim text arrays work here.
Example:
from manim import *
class text_highlight(Scene):
def construct(self):
t1 = Text("Never gonna")
t2 = Tex("Give ", "You ", "up").scale(1.2)
g = VGroup(t1, t2)
g.arrange(DOWN, aligned_edge = LEFT)
self.play(Write(t1), Write(t2))
self.play(Indicate(t1[:5]))
self.play(Indicate(t2[0]))
self.play(Circumscribe(t1[5:10]))
self.play(Circumscribe(t2[2]))
self.wait()
Output:
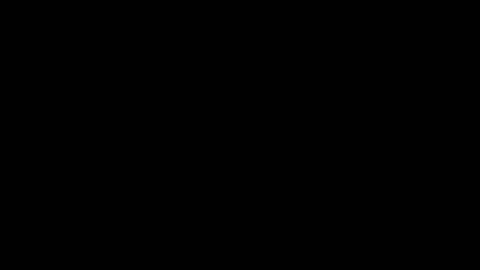
Code explanation:
Line 1: I imported the Manim library into VS Code.
Lines 3 and 4: I created a new Manim scene and named it “text_highlight”.
Line 5: I created a new Text() that said “Never gonna” and assigned it to a new variable that I named t1.
Line 6: I created a new Tex() (which is text formatted in LaTeX) that said “Give you up” and assigned it to a new variable that I named t2. I also separated each word with a comma which separated these words inside of the array as separate objects.
Line 7: I grouped both pieces of text using a VGroup() and assigned the group to a new variable that I named g.
Line 8: I arranged the objects in the group from top to bottom and aligned them to the left edge. You can learn more about how to align objects in Manim here.
Line 10: I animated both pieces of text on the screen at the same time using the Write() animation type for each.
Line 11: I highlighted the word “Never” using the Indicate() command. If you use the Text() command to create your text, then every letter is a separate object inside of the array. You can learn more about text arrays in Manim here.
Line 12: I highlighted the word “Give” using the Indicate() command. If you use the Tex() command to create your text, then every word that you separate with a comma acts as a separate object inside of the array. That’s why when I said self.play(Indicate(t2[0])) Manim highlighted the object number 0 in the array, which was the word “Give”.
Line 13: I highlighted the word “gonna” using the Circumscribe() command. Since I created the text “Never gonna” using the Text() command, every letter in that piece of text was a separate object in the Python array.
Line 14: I highlighted the word “up” using the Circumscribe() command. Since I created this text using the Tex() command, every word that I separated with a comma was a separate object in the Python array. Also, keep in mind that objects in Python arrays start from the number 0. So the first object will always have the index 0.
Line 15: I paused the animation on the screen for 1 second.
Let me help you learn Manim
If you want to skip the headache of trying to learn Manim from a bunch of scattered information, I put together a comprehensive 3-hour Manim course for complete beginners.
It will give you all the foundational skills you need to start creating stunning animations with code.
Enroll In Manim Course For Beginners