There are a few different methods that you can use to align text or other elements next to each other in Manim.
Table of contents:
- How to left align text in Manim
- How to position objects next to each other in Manim
- How to align objects using a VGroup
How to left align text in Manim
To align multiple Text objects to the left side put them inside of a VGruop and then align all of the objects inside that group.
Here’s an example:
from manim import *
class text(Scene):
def construct(self):
t1 = Text("We're no strangers to love")
t2 = Text("You know the rules and so do I")
g = VGroup(t1, t2)
g.arrange(DOWN, aligned_edge = LEFT)
self.play(Write(t1))
self.play(Write(t2))
self.wait(3)
Output:
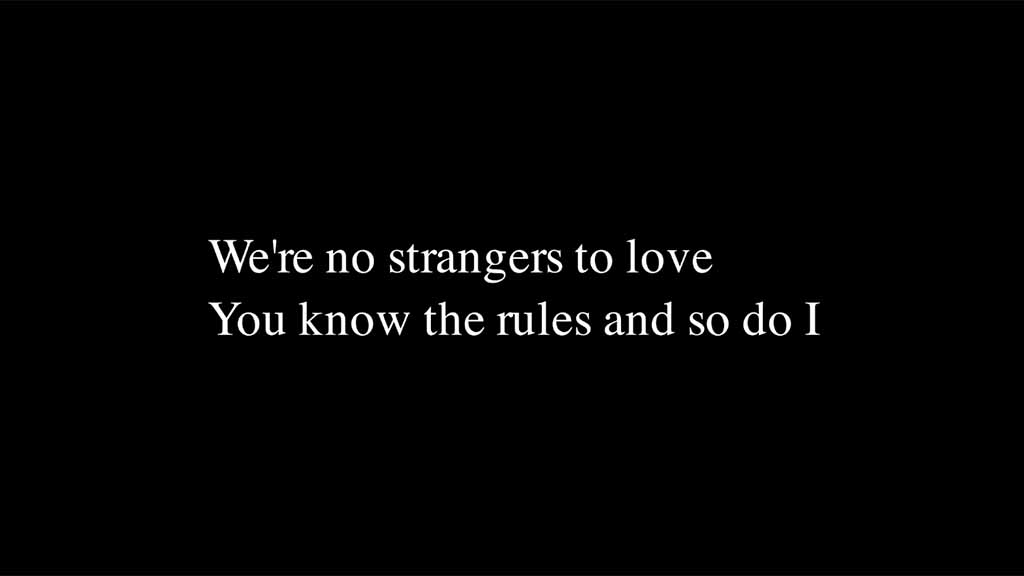
As you can see the text is aligned to the left edge.
I’ll explain what each line of the code does:
Line 1: I imported Manim into vscode so that it understands I’m writing Manim code.
Lines 3 and 4: I created a new scene called “text”. Each new scene in your Python file will output a separate animation file. You can create as many scenes inside of your project as you want to.
Lines 5 and 6: I created two new Text objects and assigned them to variables t1 and t2.
Line 7: I grouped both of the new Text objects using a VGroup (vector group). Grouping objects allows you to animate them together.
Line 8: I arranged all objects inside of the VGroup down and aligned them to the left edge.
Lines 9 and 10: I told Manim that I wanted to animate both t1 and t2 on the screen using the Write animation type.
Line 11: I told Manim that I wanted to pause the animation for 3 seconds after it’s finished.
I recommend checking out this guide about Manim Text – How To Move, Transform, Change, And Animate Text.
How to position objects next to each other in Manim
To position mobjects next to each other in Manim you can use the next_to() command.
Example:
from manim import *
class next_to(Scene):
def construct(self):
c = Circle()
s = Square().next_to(c, UP)
r = RegularPolygon(n = 8).next_to(s, RIGHT)
t = Triangle().next_to(r, DOWN*10)
self.play(Create(c), Create(s), Create(r), Create(t))
self.wait(3)
Output:
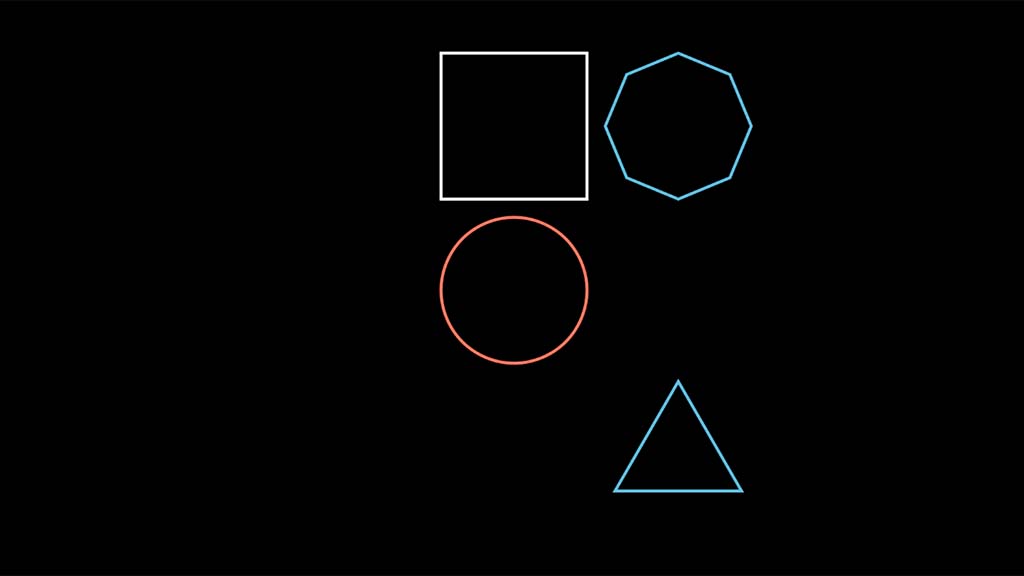
Explanation of each line of code:
Line 1: I imported the Manim library into vscode so that it understands the Manim syntax.
Lines 3 and 4: I created a new scene and named it “next_to”.
Line 5: I created a circle and assigned it to a variable that I named c.
Line 6: I created a square and assigned it to a variable that I named s. Then I said that I wanted to position this square at the top of the circle.
Line 7: I created a polygon with 8 sides (n indicates how many sides it should have) and assigned it to a variable that I named r. Then I said that I wanted to position this polygon next to the square on the right side.
Line 8: I created a triangle and assigned it to a variable that I named t. Then I said that I wanted to position this triangle next to the polygon and at the bottom of it. I then multiplied the word “DOWN” by 10 times indicating that I want to position it at a distance that’s 10 times greater than the default distance.
Line 9: I asked Manim to animate all of these shapes on the screen using the Create() animation method.
Line 10: I asked Manim to pause the animation for 3 seconds.
How to align objects using a VGroup
One of the best ways to align objects in Manim is by putting them inside a group and then aligning that group.
1. Align objects in a VGroup using the arrange() command
To align multiple objects create a VGroup that houses all of them and then use the arrange() command to arrange all the objects within that group.
from manim import *
class groups(Scene):
def construct(self):
s = Square().scale(0.5)
t1 = Text("Hello")
t2 = Text("Ladies")
t3 = Text("And")
t4 = Text("Mentlegen")
gr = VGroup(s, t1, t2, t3, t4)
gr.arrange(DOWN, aligned_edge = LEFT)
self.play(Write(gr))
self.wait(3)
Output:
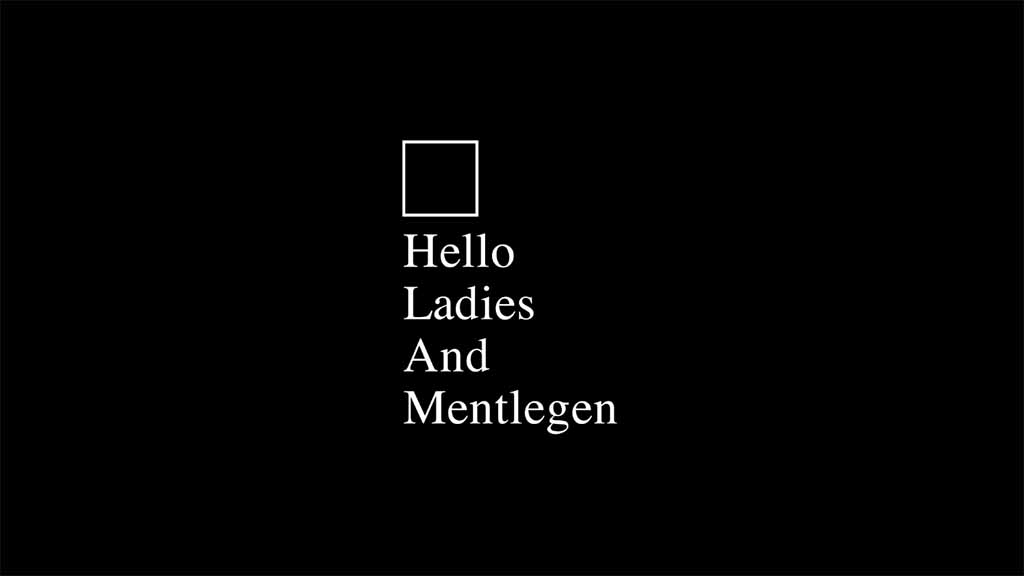
Explanation of each line of code:
Line 1: I imported Manim into VS Code.
Lines 3 and 4: I created a new scene and called it “groups”.
Line 5: I created a square, scaled it down to 50%, and assigned it to a new variable that I called s.
Line 6, 7, 8, and 9: I created 4 pieces of text and assigned each of them to new variables called t1, t2, t3, and t4.
Line 10: I put the square variable as well as all of the text variables inside of a group and I assigned that group to a new variable that I called gr.
Line 11: I arranged all the objects that were in that group down and also aligned them to the left edge.
Line 12: I animated the group on the screen by using the self.play() command and the Write() animation type.
Line 13: I paused the animation on the screen for 3 seconds.
If you only used the arrange() command with empty parentheses it would have arranged all submobjects in that group left to right and put the center of all of those objects in the center of the frame.
2. Align objects in a VGroup to a grid using the arrange_in_grid() command
Example:
from manim import *
class triangles(Scene):
def construct(self):
triangles = VGroup(
*[Triangle().scale(0.2) for i in range(70)]
)
triangles.set_color_by_gradient(RED, ORANGE, YELLOW)
triangles.arrange_in_grid(rows = 7 )
self.play(Write(triangles))
self.wait(3)
Output:
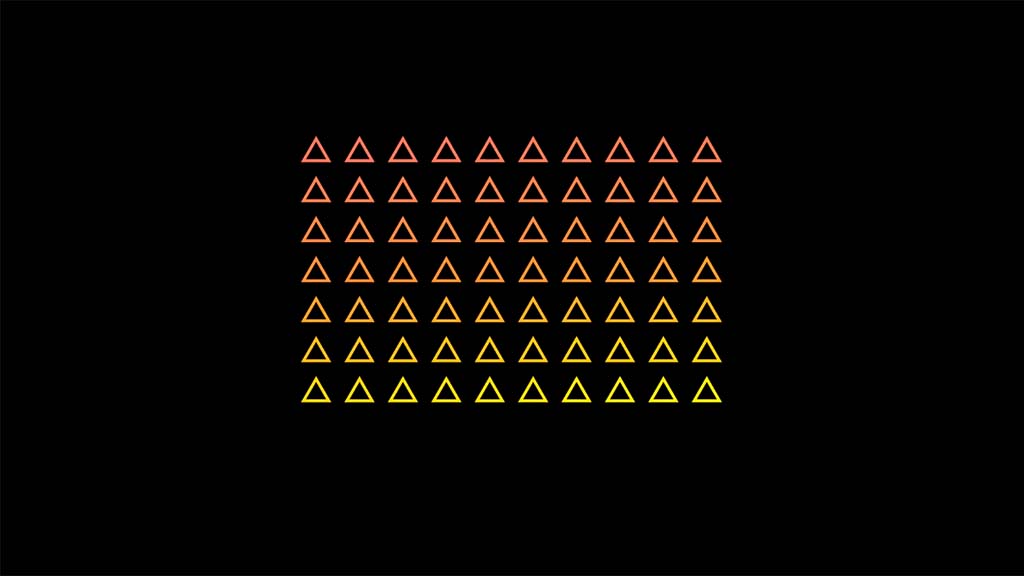
Explanation of each line of code:
Line 1: I imported the Manim library into my Python file within VS Code.
Lines 2 and 3: I created a new scene and called it “triangles”.
Line 5: I created a VGroup and assigned it to a new variable that I called triangles. Inside of this VGroup, I will be creating the triangles so that I don’t have to put them in a group later when I’ll want to arrange them in the grid pattern.
Line 6: I created 70 triangles and scaled them to 20% of their original size by using a Python for loop.
Line 8: Now that I had all the triangles inside of a group I thought why not make them look nicer by coloring them with a gradient? So I set the gradient for the group of triangles to go from color red to orange and finally to yellow.
Line 9: I arranged the group with 70 triangles in a grid which I said should have 7 rows. Since I had 70 triangles arranging them in 7 rows meant that every single row had the same amount of them.
Line 10: I animated the triangle group on the screen using the Write() animation type.
Line 12: I paused the animation for 3 seconds.
Let me help you learn Manim
If you want to skip the headache of trying to learn Manim from a bunch of scattered information, I put together a comprehensive 3-hour Manim course for complete beginners.
It will give you all the foundational skills you need to start creating stunning animations with code.
Enroll In Manim Course For Beginners