To change the animation play time in Manim use the run_time parameter inside of the self.play() command.
By default, each animation in Manim lasts 1 second.
How to change the speed of an animation in Manim
You can use the run_time parameter to change how long a Manim animation plays on the screen.
Example:
from manim import *
class speed(Scene):
def construct(self):
c = Circle()
s = Square()
self.play(Write(c))
self.play(ReplacementTransform(c, s), run_time = 5)
self.play(Unwrite(s), run_time = 0.5)
Output:
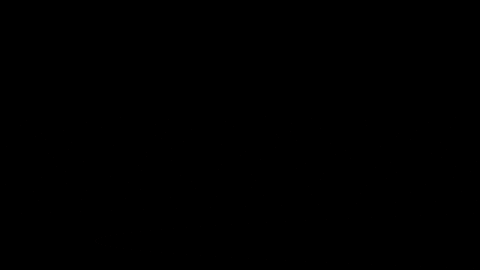
Code explanation:
Line 1: I imported the Manim library into Python. This allows VS Code to understand Manim code syntax.
Lines 3 and 4: I created a new scene and named it “speed”.
Line 5: I created a circle and assigned it to a new variable that I named c.
Line 6: I created a square and assigned it to a new variable that I named s.
Line 7: I animated the circle on the screen using the Write() animation type.
Line 8: I transformed the circle into the square using ReplacementTransform(). I wanted to change this animation duration from the default 1 second to 5 seconds so after a comma, I added the run_time = 5 parameter. This makes all the animations inside of the self.play() play over the duration that you define.
Line 9: I removed the square from the screen by using the Unwrite() animation and also changed its play time to 0.5 seconds using the run_time parameter.
How to animate objects in Manim at the same time but with different speed
You can animate different mobjects within the same self.play() command at different speeds by putting the run_time parameter inside the parentheses of each animation function that you’re using to animate those objects.
Example:
from manim import *
class speed2(Scene):
def construct(self):
d1 = Dot(color = GREEN)
d2 = Dot(color = RED)
d3 = Dot(color = BLUE)
g = VGroup(d1, d2, d3).scale(10)
g.arrange()
self.play(
Write(d1, run_time = 1.5),
Write(d2, run_time = 3),
Write(d3, run_time = 4.5)
)
Output:
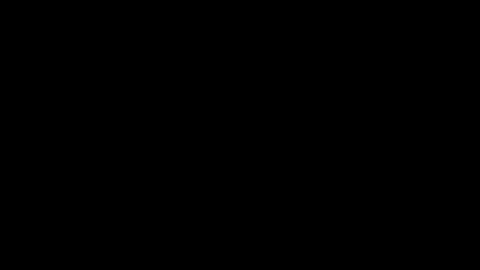
Code explanation:
Line 1: I imported the Manim library into Python.
Lines 3 and 4: I created a new scene and named it “speed2”.
Lines 5, 6, and 7: I created 3 dots, each in a different color, and assigned each of them to a new variable d1, d2, and d3.
Line 9: I put all three dots into a VGroup(). This allowed me to scale all of the dots at the same time. So I added the .scale() parameter and scaled them to 10x their original size.
Line 10: I arranged the dot group by using the .arrange() command and left the parentheses empty. This arranged everything in the group using the default settings (left to right and aligned to the center). You can read more about how to align objects in Manim here.
Lines 12 to 16: I animated all of the dots on the screen using different animation play times for each one. I picked the Write() animation type to animate all of them and then, inside the parentheses of each Write() command, I put a comma and specified the run_time for each of them.
Let me help you learn Manim
If you want to skip the headache of trying to learn Manim from a bunch of scattered information, I put together a comprehensive 3-hour Manim course for complete beginners.
It will give you all the foundational skills you need to start creating stunning animations with code.
Enroll In Manim Course For Beginners