There are so many different ways to animate objects inside of the self.play() command in Manim.
When I started learning Manim I was eager to discover all of them because each one looked super cool.
So here are some cool animations (with cool additional attributes) you can add to your Manim animations:
- ReplacementTransform
- Transform
- TransformMatchingShapes
- Write (Unwrite)
- Swap
- Restore
- Create (Uncreate)
- Circumscribe
- Indicate
- GrowFromCenter
- DrawBorderThenFill
- FadeToColor
- FadeTransform
- ShowCreationThenFadeOut
- CyclicReplace
- FocusOn
- SpiralIn
- ApplyWave
- Flash
- Wiggle
- Broadcast
To see all available animations in Manim visit the Manim Community website.
ReplacementTransform
Example:
from manim import *
class animations(Scene):
def construct(self):
t = Text("ReplacementTransform")
s = Square(color = RED)
self.play(Write(t))
self.wait()
self.play(ReplacementTransform(t, s))
self.play(s.animate.scale(2))
self.wait(2)
Output:
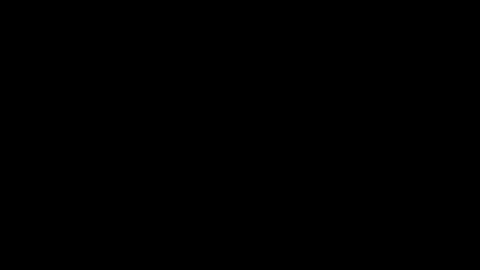
Additional attributes:
path_arc – sets the arc angle (in radians, not degrees) that the points of the first object will follow to reach the points of the second object.
If I change the 9th line to this:
self.play(ReplacementTransform(t, s, path_arc = PI+1))
This is the output:
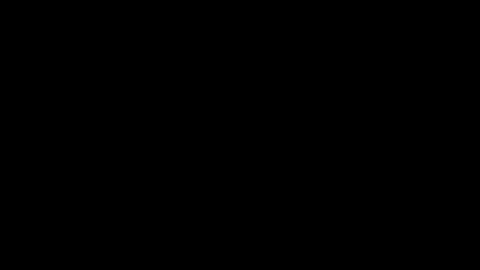
Transform
Example:
from manim import *
class animations(Scene):
def construct(self):
t = Text("Transform")
s = Square(color = RED)
self.play(Write(t))
self.wait()
self.play(Transform(t, s))
self.play(s.animate.scale(2))
self.wait(2)
Output:
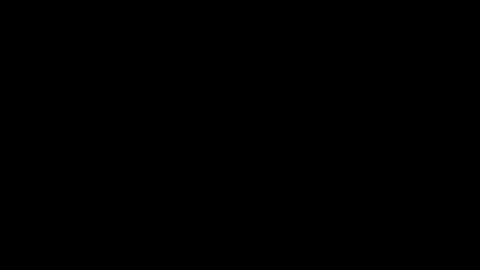
The difference between ReplacementTransform and Transform in Manim is that ReplacementTransform replaces and morphs a mobject into another mobject and Transform morphs a mobject into another mobject but doesn’t replace it.
TransformMatchingShapes
Example:
from manim import *
class animations(Scene):
def construct(self):
t1 = Text("TransformMatchingShapes")
t2 = Text("What are you doing in my swamp?")
self.play(Write(t1))
self.wait()
self.play(TransformMatchingShapes(t1, t2))
self.wait(2)
Output:
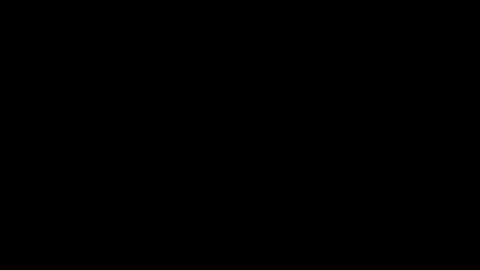
Additional attributes:
transform_mismatches = True – will transform the other letters into new ones without fading them into place (I think it looks cooler when this option is set to True).
Here’s what it looks like with it set to True:
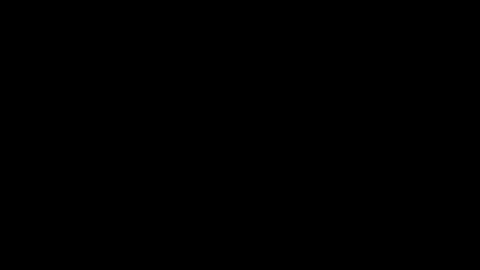
fade_transform_mismatches = True – will transform the non-matching letters using the FadeTransform animation instead of the default Transform animation.
Write (Unwrite)
Example:
from manim import *
class animations(Scene):
def construct(self):
t = Text("I like this one")
self.play(Write(t))
self.wait()
self.play(Unwrite(t))
Output:
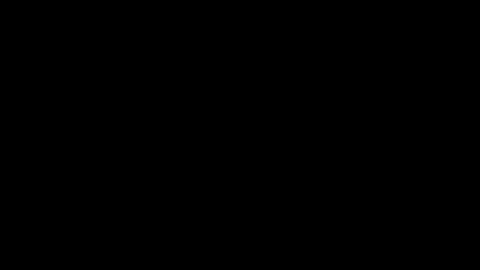
Additional attribute:
reverse = True – writes the text from the right edge.
Swap
Example:
from manim import *
class animations(Scene):
def construct(self):
c = Circle()
p = RegularPolygon()
g = VGroup(c, p).arrange(buff = 1)
self.play(Write(c), Write(p))
self.wait(0.1)
self.play(Swap(c, p))
self.wait(0.5)
Output:
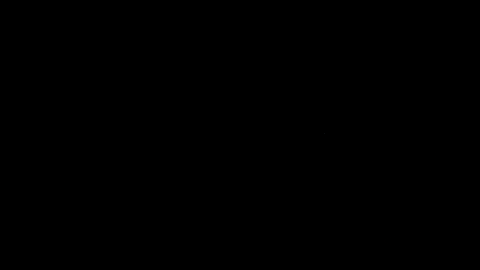
Additional attributes:
path_arc – sets the angle (in radians, not degrees) that the objects will follow as they swap places.
Restore
Example:
from manim import *
class animations(Scene):
def construct(self):
c = Circle(color = GREEN, fill_opacity = 1)
c.save_state()
self.play(c.animate.scale(6))
self.play(c.animate.shift(RIGHT*4))
self.play(FadeToColor(c, RED))
self.play(Restore(c))
self.wait()
Output:
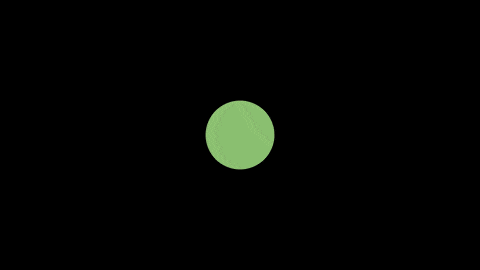
Create (Uncreate)
Example:
from manim import *
class animations(Scene):
def construct(self):
c1 = Circle(color = GREEN)
c2 = Circle(color = RED)
c3 = Circle(color = BLUE)
g = VGroup(c1, c2, c3).arrange()
self.play(Create(c1), Create(c2), Create(c3))
self.wait()
self.play(Uncreate(g))
self.wait(0.5)
Output:
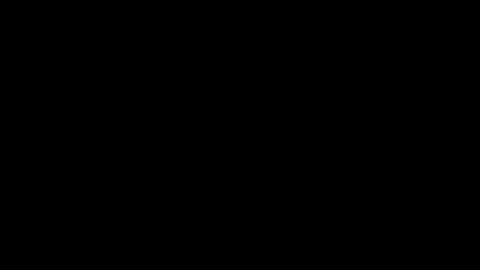
Circumscribe
from manim import *
class animations(Scene):
def construct(self):
t = Text("Shrek", color = RED, font = "Sentient").scale(2)
self.play(Write(t))
self.play(Circumscribe(t))
self.wait(0.5)
Output:
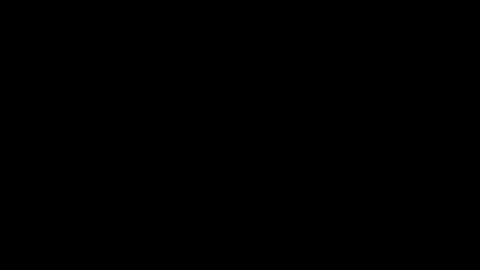
Additional attributes:
Just like shapes in Manim, the Circumscribe rectangle has similar attributes to customize how it looks like.
color – sets the color of the outline.
stroke_width – sets how thick the shape’s stroke width (the outline) is.
shape = Circle – changed the highlighting shape from a rectangle to a circle.
buff – sets the space between the rectangle and the object that it’s being drawn around.
time_width – sets the time gap between the drawing and undrawing of the rectangle
Here’s an example with all of these attributes applied:
from manim import *
class animations(Scene):
def construct(self):
t = Text("Shrek", color = RED, font = "Sentient").scale(2)
self.play(Write(t))
self.play(Circumscribe(t, color = BLUE, stroke_width = 50, shape = Circle, buff = 1.4, time_width = 3))
self.wait(0.5)
Output:
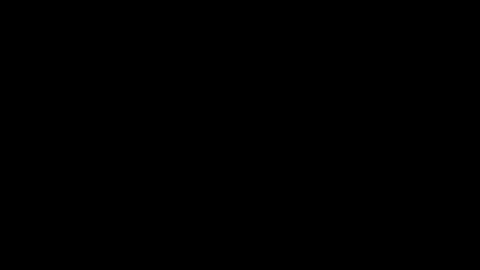
Indicate
from manim import *
class animations(Scene):
def construct(self):
t = Text("Shrek", color = RED, font = "Sentient").scale(2)
self.play(Write(t))
self.play(Indicate(t))
self.wait(0.5)
Output:
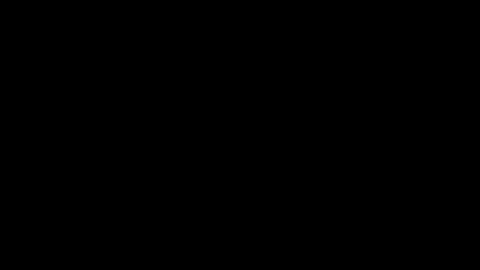
Additional attributes:
scale_factor – sets how big (or small) the indication gets (the default value is 1.2).
color – sets the color of the indication (the default value is YELLOW).
GrowFromCenter
Example:
from manim import *
class animations(Scene):
def construct(self):
s = RoundedRectangle(color = BLUE, stroke_width = 10, corner_radius = 0.5, width = 2, height = 2)
ss = SurroundingRectangle(s, color = ORANGE, corner_radius = 0.5, buff = 1.5, stroke_width = 10)
g = VGroup(s, ss)
self.play(GrowFromCenter(g))
self.wait(0.5)
Output:
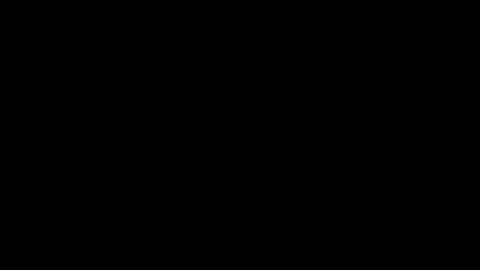
Additional attribute:
point_color – sets the initial color of the mobject before it grows to its full size.
In this example, I set the point_color to RED, which means that when the shape starts growing it will turn from RED to its original color (which is BLUE in this case):
from manim import *
class animations(Scene):
def construct(self):
r = Square(color = BLUE, fill_opacity = 1).scale(2)
self.play(GrowFromCenter(r, point_color = RED), run_time = 2)
self.wait(0.5)
Output (the gif compression makes the colors look a little weird):
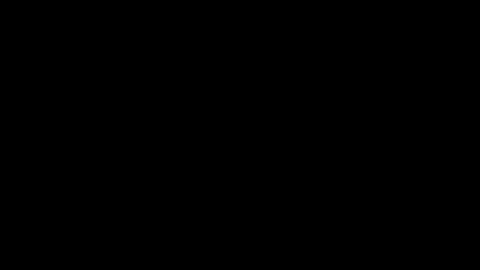
DrawBorderThenFill
Example:
from manim import *
class animations(Scene):
def construct(self):
t = Triangle(color = RED, fill_opacity = 1).scale(2)
self.play(DrawBorderThenFill(t))
self.wait(0.5)
Output:
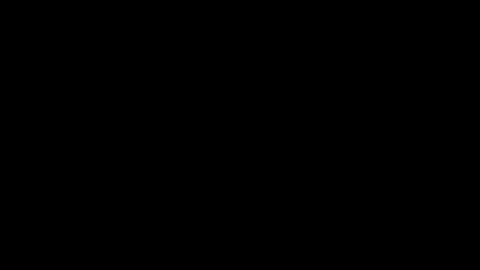
Additional attributes:
stroke_color – sets the stroke’s color with which the object’s outline will be drawn. Then the stroke’s color will fade to the object’s original stroke color.
stroke_width – sets the stroke width with which the object’s outline will be drawn. Then the stroke’s width will transform into the object’s original stroke width.
Example:
from manim import *
class animations(Scene):
def construct(self):
t = Circle(color = RED, stroke_color = WHITE, fill_opacity = 1).scale(2)
self.play(DrawBorderThenFill(t, stroke_color = BLUE, stroke_width = 30))
self.wait(0.5)
Output:
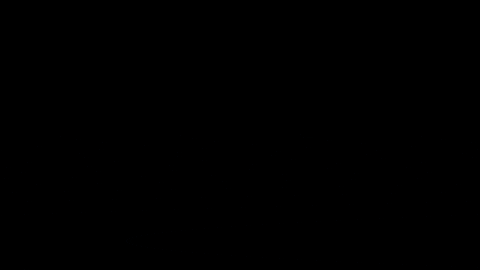
FadeToColor
Example:
from manim import *
class animations(Scene):
def construct(self):
c = Circle(color = RED, fill_opacity = 1).scale(2)
self.play(Write(c))
self.play(FadeToColor(c, BLUE))
self.wait(0.5)
Output:
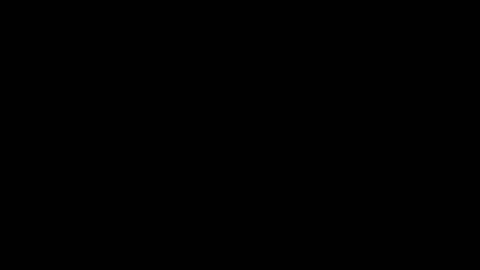
FadeTransform
Example:
from manim import *
class animations(Scene):
def construct(self):
c = Circle(color = RED, fill_opacity = 1)
r = Square(color = BLUE).scale(2)
self.play(Write(c))
self.play(FadeTransform(c, r))
self.wait(0.5)
Output:
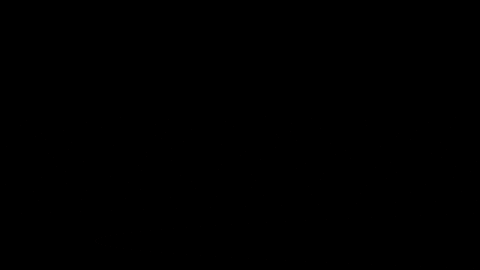
Additional attribute:
stretch = False – controls whether the target Mobject is stretched during the animation (the default value is set to True).
stretch = True vs stretch = False:
from manim import *
class animations(Scene):
def construct(self):
c1 = Circle(color = RED, fill_opacity = 1)
r1 = Rectangle(color = BLUE)
t1 = Text("stretch = True")
g1 = VGroup(c1, r1)
c2 = Circle(color = RED, fill_opacity = 1)
r2 = Rectangle(color = BLUE)
t2 = Text("stretch = False")
g2 = VGroup(c2, r2)
gg = VGroup(g1, g2).arrange(buff = 1)
t1.next_to(r1, UP)
t2.next_to(r2, UP)
self.add(c1, c2, t1, t2)
self.play(FadeTransform(c1, r1), FadeTransform(c2, r2, stretch = False))
self.play(FadeTransform(r1, c1), FadeTransform(r2, c2, stretch = False))
self.wait(0.5)
Output:
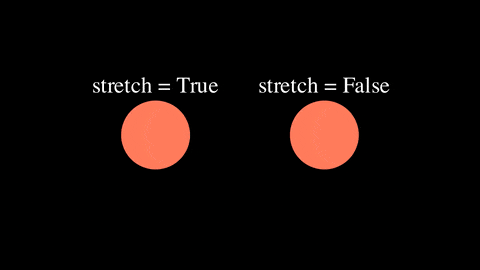
ShowCreationThenFadeOut
Example:
from manim import *
class animations(Scene):
def construct(self):
c = Circle(color = RED, stroke_width = 10)
self.play(ShowCreationThenFadeOut(c))
self.wait(0.5)
Output:
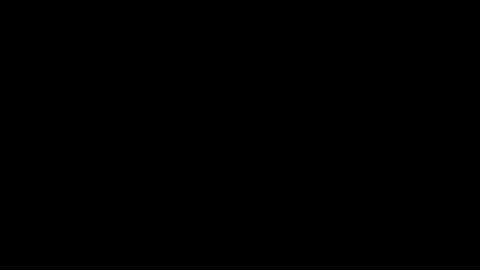
CyclicReplace
Example:
from manim import *
class animations(Scene):
def construct(self):
d1 = Dot(color = RED)
d2 = Dot(color = GREEN)
d3 = Dot(color = BLUE)
g = VGroup(d1, d2, d3).arrange().scale(10)
self.play(Write(g))
self.play(CyclicReplace(d1, d2))
self.play(CyclicReplace(d2, d3))
self.play(CyclicReplace(d1, d3))
self.play(CyclicReplace(d1, d2, d3))
self.play(CyclicReplace(d3, d1, d2))
self.play(Unwrite(g))
Output:
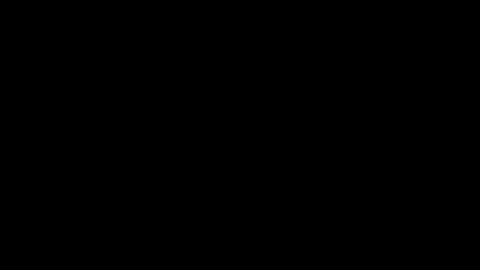
path_arc – sets the angle of the arc (in radians) that the objects will follow. Essentially, how big of a circle each object draws when they move into place.
FocusOn
Example:
from manim import *
class animations(Scene):
def construct(self):
t1 = Text("Hello", color = RED, font = "Sentient").scale(2)
t2 = Text("There", color = RED, font = "Sentient").scale(2)
g = VGroup(t1, t2).arrange(buff = 2)
self.play(Write(g))
self.play(FocusOn(t2))
self.wait(0.5)
Output:
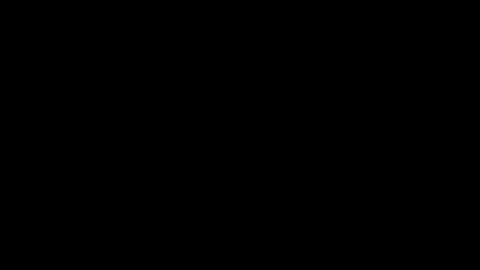
Additional attributes:
opacity – sets how visible the focus circle is (the default value is set to 0.2).
color – sets the color of the focus circle (the default value is set to GREY).
SpiralIn
Example:
from manim import *
class animations(Scene):
def construct(self):
t = Text("Shrek", color = RED, font = "Sentient").scale(2)
s = Square()
self.play(SpiralIn(t))
self.wait(0.5)
self.play(Unwrite(t))
self.wait(0.5)
Output:
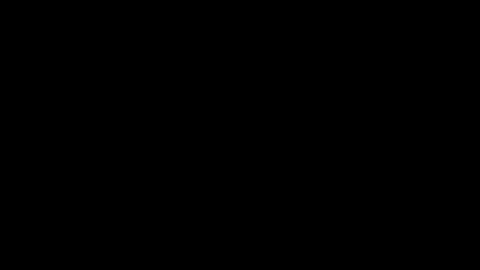
Additional attributes:
scale_factor – sets how big the object is once it starts spiraling in on the screen (the default value is set to 8).
fade_in_fraction – sets how long the sub-mobjects will fade as they spiral in (the default value is set to 0.3).
ApplyWave
Example:
from manim import *
class animations(Scene):
def construct(self):
t = Text("Shrek", color = RED, font = "Sentient").scale(2)
self.play(Write(t))
self.play(ApplyWave(t))
self.wait(0.5)
Output:
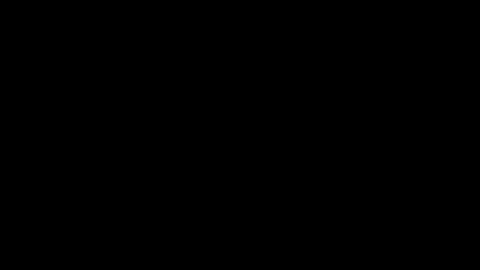
Additional attributes:
direction – sets the direction in which the wave moves the points of the shape (default: UP).
amplitude – sets how tall the wave gets (default: 0.2).
time_width – sets the length of the wave relative to the width of the mobject (default: 1).
ripples – sets the amount of wave ripples (default: 1).
Example with all additional attributes applied:
from manim import *
class animations(Scene):
def construct(self):
t = Text("Shrek", color = RED, font = "Sentient").scale(2)
self.play(Write(t))
self.play(ApplyWave(t, direction = LEFT, amplitude = 0.3, time_width = 2, ripples = 3))
self.wait(0.5)
Output:
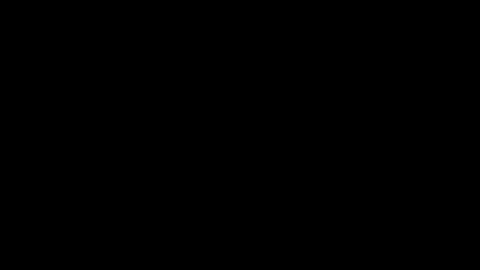
Flash
Example:
from manim import *
class animations(Scene):
def construct(self):
d1 = Dot(point = ([-4, 3, 0]))
d2 = Dot(point = ([4, -3, 0]))
self.play(Write(d1), Write(d2))
self.wait(0.5)
self.play(Flash(d1, line_length = 0.5))
self.wait(0.5)
Output:
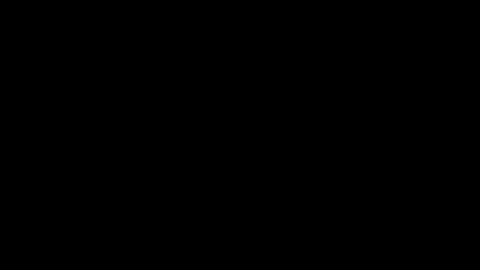
Additional attributes:
point – sets the center of the flash. If it’s is a mobject then the flash will start in the center of it. See Manim coordinate system.
line_length – sets the length of the flash lines (default: 0.2).
num_lines – sets the number of flash lines (default: 12).
flash_radius – sets the distance from the point at which the flash lines start (default: 0.1).
line_stroke_width – sets how thick the lines are (default: 3).
color – sets the color of the lines (default: YELLOW).
time_width – sets how long the line stays on the screen before it starts shrinking (default: 1).
Example with additional attributes applied:
from manim import *
class animations(Scene):
def construct(self):
self.play(Flash([-3, 1, 0], line_length = 1, num_lines = 30, flas_radius = 0.9, line_stroke_width = 1, color = RED, time_width = 0.2))
self.play(Flash([3, -1, 0], line_length = 5, num_lines = 15, flas_radius = 0.5, line_stroke_width = 5, color = RED, time_width = 0.5))
self.wait(0.5)
Output:
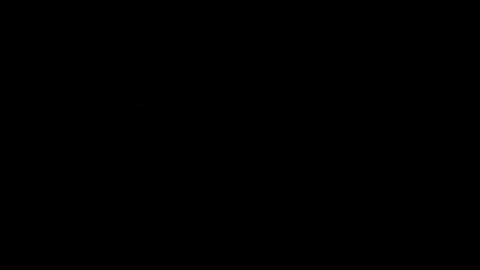
Wiggle
Example:
from manim import *
class animations(Scene):
def construct(self):
s = Square(color = RED, fill_opacity = 0.5, fill_color = BLUE)
self.play(Write(s))
self.wait(0.5)
self.play(Wiggle(s))
self.wait(0.5)
self.play(Unwrite(s))
Output:
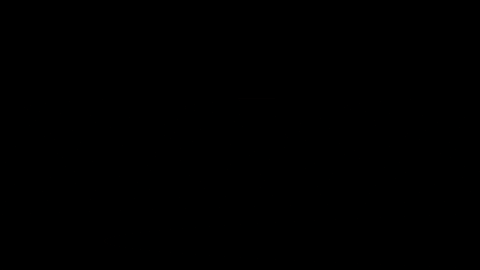
Additional attributes:
scale_value – sets how big the mobject will get (default: 1.1).
rotation_angle – sets how much the object will rotate (default: 0.01).
n_wiggles – sets the number of wiggles (default: 6).
scale_about_point – sets the point about which the mobject gets scaled.
rotate_about_point – sets the point around which the mobject gets rotated.
Example with additional attributes applied:
from manim import *
class animations(Scene):
def construct(self):
s = Square(color = RED, fill_opacity = 0.5, fill_color = BLUE)
self.add(s)
self.play(Wiggle(s, scale_value = 1.5, rotation_angle = 0.5, n_wiggles = 3, scale_about_point = s.get_corner(UL), rotate_about_point = s.get_corner(DR)))
self.wait(0.5)
Output:
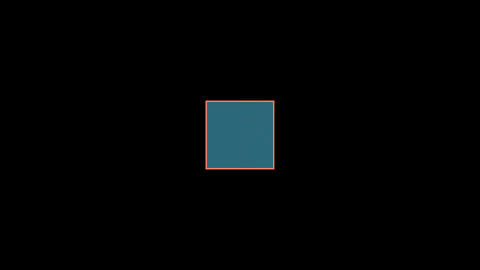
Broadcast
Example:
from manim import *
class animations(Scene):
def construct(self):
s = Square(color = BLUE, fill_opacity = 0.5, fill_color = RED).scale(2)
self.play(Write(s))
self.wait(0.5)
self.play(Broadcast(s))
self.wait(0.5)
self.play(Unwrite(s))
Output:
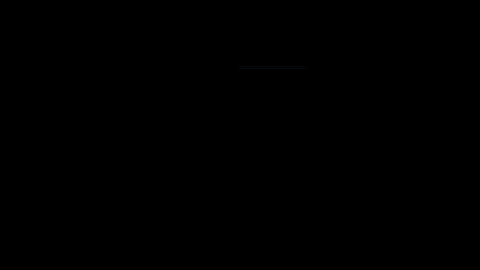
Additional attributes:
focal_point – sets the center of the broadcast (default: ORIGIN).
n_mobs – sets the number of mobjects that emerge from the focal point (default: 5).
initial_opacity – sets the starting stroke opacity of the mobjects that get broadcasted (default: 1).
final_opacity – sets the final stroke opacity of the mobjects that get broadcasted (default: 0).
initial_width – sets the initial width of the mobjects (default: 0).
remover – sets whether the mobjects should be removed from the scene after the animation (default: True).
Example with some additional attributes applied:
from manim import *
class animations(Scene):
def construct(self):
s = Square(color = BLUE, fill_opacity = 0.5, fill_color = RED)
self.play(Write(s))
self.wait(0.5)
self.play(Broadcast(s, focal_point = s.get_corner(UL), n_mobs = 3, initial_opacity = 0.5, final_opacity = 1, remover = False))
self.wait(0.5)
Output:
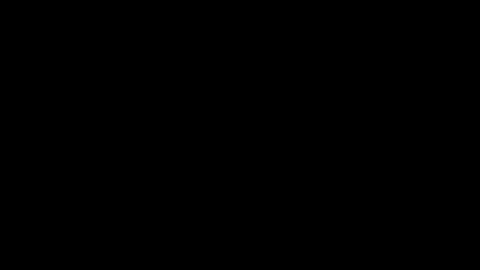
Let me help you learn Manim
If you want to skip the headache of trying to learn Manim from a bunch of scattered information, I put together a comprehensive 3-hour Manim course for complete beginners.
It will give you all the foundational skills you need to start creating stunning animations with code.
Enroll In Manim Course For Beginners