You can create multiple objects with a loop in Manim and add all of those objects into a VGroup() automatically like this:
g = VGroup(*[Dot() for i in range(100)])
This will create 100 Dots and add them into a VGroup().
You can then arrange them however you want by arranging the group:
g.arrange_in_grid()
Example #1:
from manim import *
class loops(Scene):
def construct(self):
g = VGroup(*[Dot() for i in range(100)])
g.arrange_in_grid(rows = 10)
self.play(Write(g))
Output:
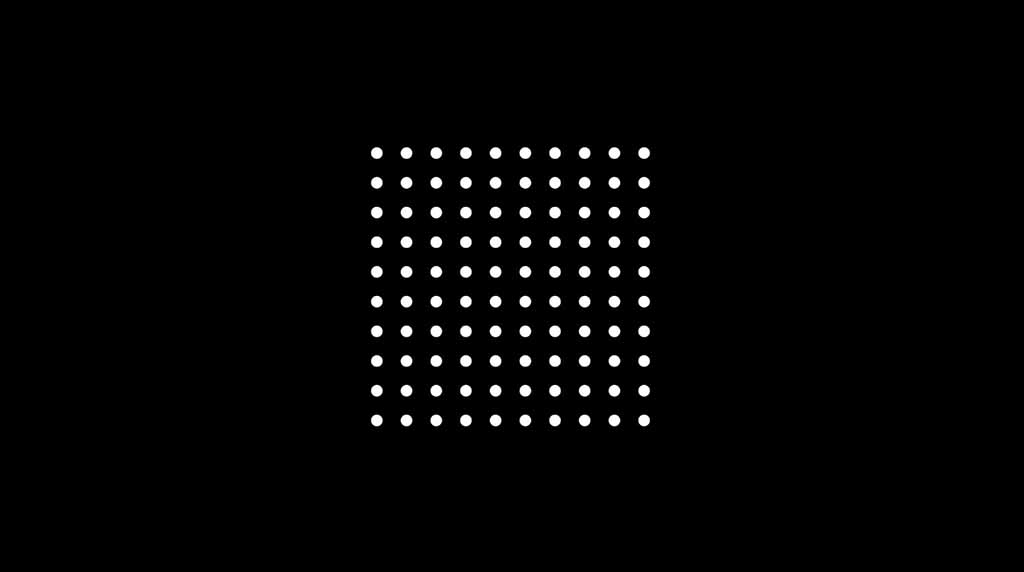
Code explanation:
Line 1: I imported the Manim library into VS Code.
Lines 3 and 4: I created a new Manim scene named “loops”.
Line 5: I created a VGroup() and assigned it to a new variable g. Inside the group’s parentheses, I created 100 dots with a loop.
Line 6: I arranged all of the objects in the group (g) in a grid and defined that the grid should have 10 rows.
Line 7: I animated all of the dots on the screen using the Write() animation type.
Example #2:
from manim import *
class loops(Scene):
def construct(self):
for i in range(10):
d = Dot(color = random_color(), sheen_factor = -0.5).scale(30-i*3)
self.play(Write(d))
self.wait()
Output:
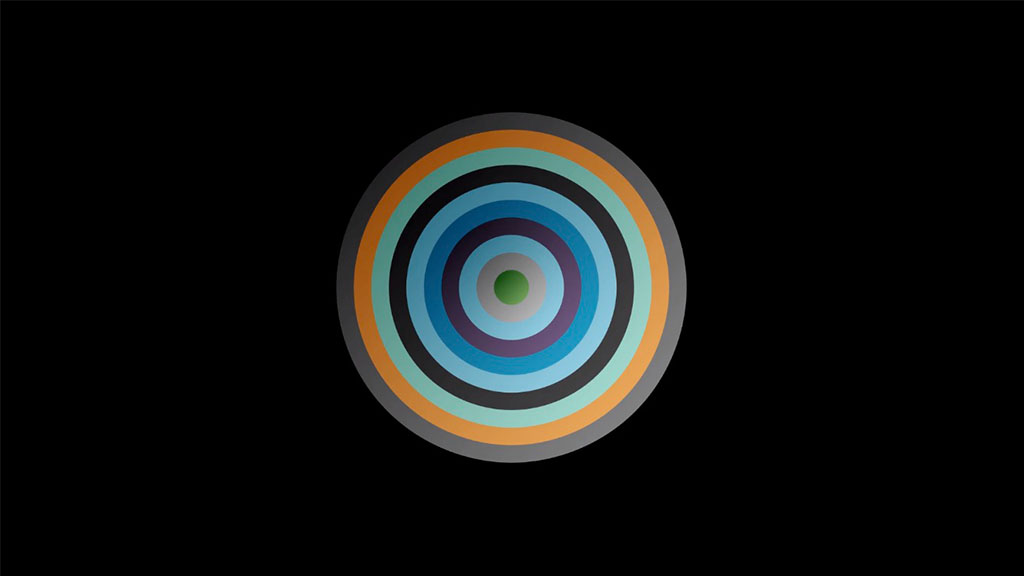
Code explanation:
Line 1: I imported the Manim library into VS Code.
Lines 3-4: I created a new scene and named it “loops”.
Lines 6-8 (inside the for loop):
- I created a new Python for loop that loops 10 times and inside of it, I created a Dot() and assigned it to a new variable I named d.
- I then said the dot should have a random color and a sheen factor of -0.5. The sheen factor makes an object shiny or if you choose a negative value it darkens one side of the object making it look more 3d.
- Then I said the dot should be scaled up 30 times minus i*3. This meant that the size of the dot was different depending on which loop it was. For example, the first time it looped around i was equal to 0 which meant that the size of the circle was 30 – 3 * 0 = 30. The fifth time it looped the size of the dot was 30 – 5*3 = 15. So as the loop looped the dots got smaller and smaller.
- Finally, for each loop, I used the Write() animation type to animate the dot on the screen.
Line 10: I paused the animation at the end for 1 second.
Example #3
Let’s try using multiple loops for creating and moving objects in Manim:
from manim import *
class loops_2(Scene):
def construct(self):
g = VGroup()
for i in range(1, 12):
s = Square(fill_opacity = 1/i, color = RED)
g.add(s)
self.play(Create(s), s.animate.shift(LEFT*6).shift(RIGHT*(i)), run_time = 0.5)
g2 = VGroup()
for i in range(len(g)):
t = Triangle(color = BLUE).move_to(g[i].get_center()).shift(DOWN)
g2.add(t)
self.play(ReplacementTransform(g[i], t), run_time = 0.5)
g2_color = g2.copy().set_color_by_gradient(RED,ORANGE,YELLOW)
g2_color.flip()
self.play(ReplacementTransform(g2, g2_color))
self.wait()
Output:
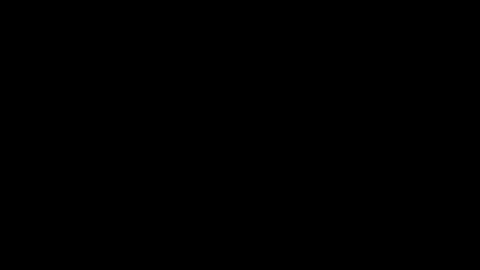
Code explanation:
Line 1: I imported the Manim library into VS Code.
Lines 3-4: I created a new Manim scene and named it “loops_2”.
Line 6: I created an empty VGroup() and assigned it to a new variable I named g. The reason it’s empty is that I’m going to be adding objects to this group after generating them with a loop.
Lines 8-11 (inside the for loop):
- I created a new Python for loop and said that it should loop for a total of 11 times (from number 1 up until 11 (the last number is not included, so the 12 doesn’t count).
- For each new loop, I created a square with the fill opacity of 1/i, which means that as the loop loops around i will increase (from 1 to 11) and the fill opacity will decrease. This is the reason I couldn’t make the loop go from i in range(0:11), instead, I did i in range(1:12) since I can’t divide 1 by 0 for the fill opacity. I also colored each square red.
- As soon as the square was created I added it to the empty VGroup() with the .add() command.
- Then I used the Create() animation type to animate each new square on the screen. I also animated the square moving to the left by 6 squares in the Manim coordinate system as well as moving right by i squares. This meant that each new square that the loop generated ended up next to each other with increasing distance from the original LEFT*6 point. I also wanted to make each animation last shorter (0.5 seconds) and I did that with the run_time() command.
Line 13: I created a new empty VGroup() once again and assigned it to a new variable named g2.
Lines 15-18 (inside the second for loop):
- I wanted to create a triangle for each square. So I made this for loop loop for the length of g, which was the group that housed all the squares I created with the previous loop.
- For each new loop, I created a new triangle, colored it blue, moved it to the center of each square by saying .move_to(g[i].get_center()) and shifted it down. For example, the first time it looped i was equal to 0, which meant that the triangle would move to the center of object g[0]. g[0] is the first square in the group that houses all the squares. So the triangle moved to the center of the first square.
- I then stored all the triangles in the second group (g2) just like I did will all the squares before.
- Finally, I animated each square in the g group transforming into each rectangle that was generated. I also said that each animation should last 0.5 seconds with the run_time() command.
Line 20: I wanted to change the color of all the rectangles so I made a copy of the second group (g2) that housed all the triangles with the .copy() command. I then assigned this copied group to a new variable I named g2_color. Finally, I colored this group with a gradient going from red to orange and to yellow by using the .set_color_by_gradient() command.
Line 21: I flipped this group horizontally with the .flip() command because I wanted to animate the non-colored triangle group (g2) flipping into the group with the colored triangles (g2_color).
Line 23: I used the ReplacementTransform() animation type to transform between the g2 group of triangles (the ones that were colored blue) into the g2_color group of triangles (which were colored with the gradient). And since I flipped the g2_color group before it added this mirror-flipping effect as it was transforming.
Line 25: I paused the animation at the end for 1 second with the self.wait() command.
Let me help you learn Manim
If you want to skip the headache of trying to learn Manim from a bunch of scattered information, I put together a comprehensive 3-hour Manim course for complete beginners.
It will give you all the foundational skills you need to start creating stunning animations with code.
Enroll In Manim Course For Beginners