You can create an arrow in Manim with the Arrow() command.
In this guide, I’ll cover everything you need to know about Manim arrows and how to animate them:
- How to create an arrow in Manim
- How to change arrow tip size and thickness in Manim
- How to point an arrow to an object
- How to create an arrow between two objects in Manim
- How to animate an arrow moving with the object in Manim
How to create an arrow in Manim
You can create an arrow in Manim with the Arrow() command.
Also, since every arrow is essentially a line with an arrow tip it’s possible to create arrows in Manim with the line() command and add an .add_tip() parameter.
Let’s look at a few examples:
Example 1:
from manim import *
class arrow(Scene):
def construct(self):
a = Arrow()
self.play(Write(a))
self.wait()
Output:
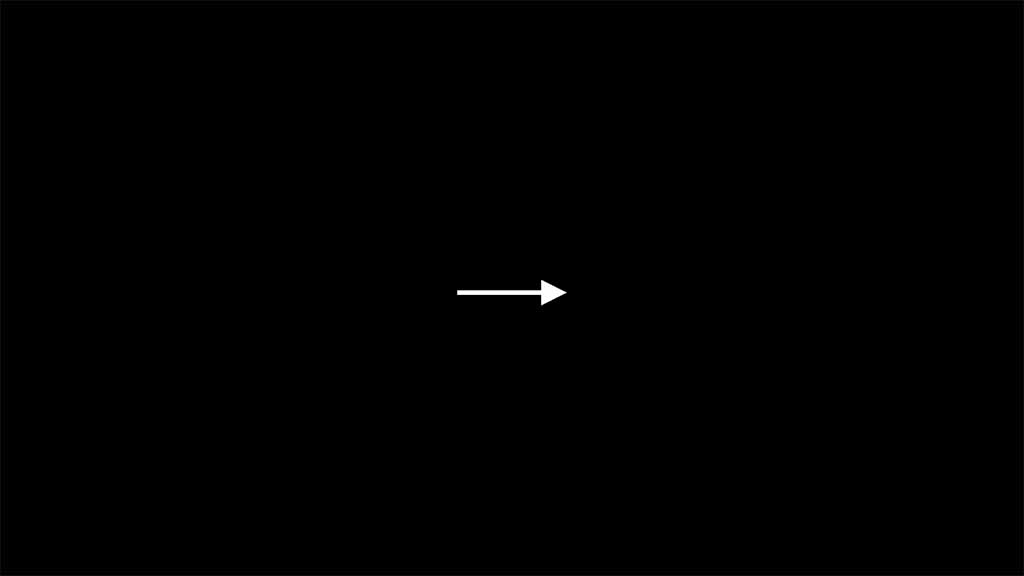
Code explanation:
Line 1: I imported the Manim library into VS Code so that Python understands Manim’s code syntax.
Lines 3 and 4: I created a new Manim scene and named it “arrow”.
Line 5: I created an arrow with the Arrow() command and assigned it to a new variable named a.
Line 6: I animated the arrow on the screen with the self.play() command using the Write() animation type.
Line 7: I paused the animation for 1 second.
Example 2:
from manim import *
class line_arrow(Scene):
def construct(self):
l = Line(start = (-2, 1, 0), end = (3, -1, 0))
l.add_tip(tip_shape = StealthTip)
self.play(Write(l))
self.wait()
Output:
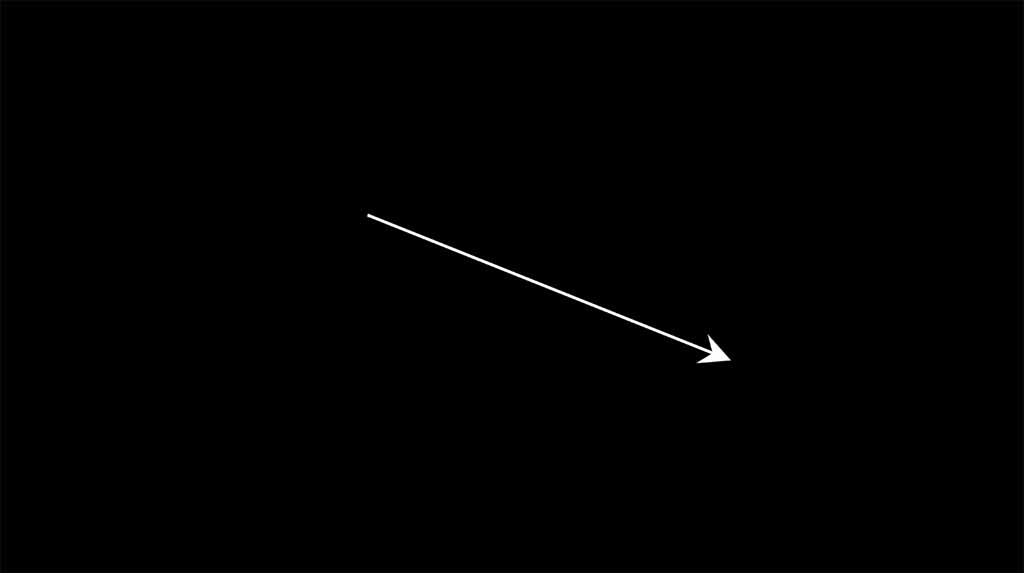
Code explanation:
Line 1: I imported the Manim library.
Lines 3 and 4: I created a new Manim scene and named it “line_arrow”
Line 5: I created a line with the Line() command. I also said that the line should start from coordinates (-2, 1, 0) and end at coordinates (3, -1, 0). Learn more about the Manim coordinate system here. The coordinates look like this (x, y, z) and z is always 0 since we’re animating in a 2d environment. And the whole scene in Manim is made up of a grid that’s 14 by 8 squares. It looks like this:
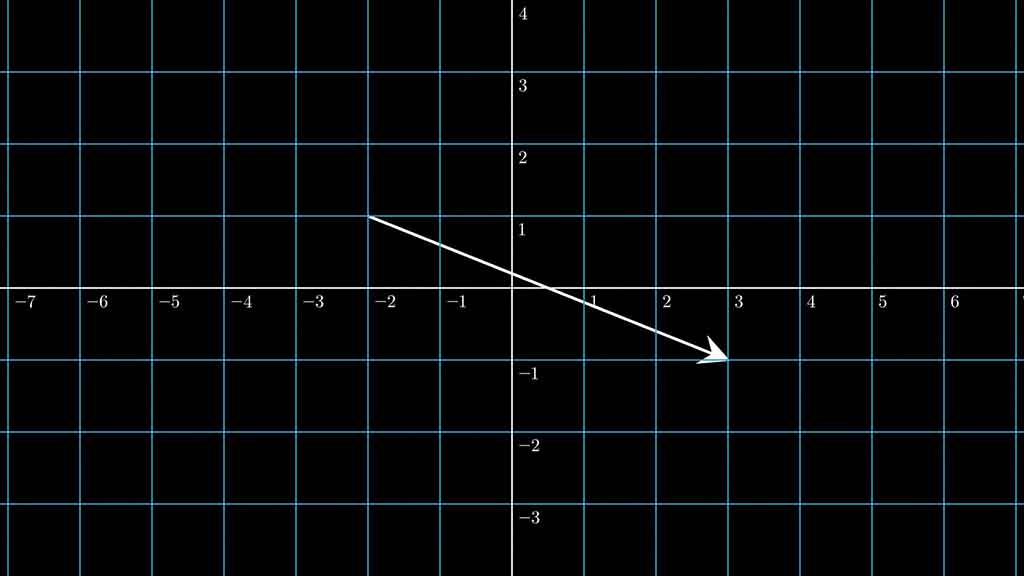
So as you can see the line goes from (-2, 1) and ends in (3, -1).
Line 6: I added the arrow tip to the line so that it looks like an arrow. I also specified the tip shape of the arrow to be the StealthTip. Otherwise, if I had left the parentheses empty the arrow shape would be default.
Line 7: I animated the line on the screen with the self.play() command using the Write() animation type.
Line 8: I paused the animation for 1 second.
How to change arrow tip size and thickness in Manim
There are a few parameters that you can use to customize your arrow in Manim.
To change the arrow tip size use the
Example:
from manim import *
class arrows(Scene):
def construct(self):
a1 = Arrow(stroke_width = 50, max_stroke_width_to_length_ratio = 100)
a1.scale(3, scale_tips = True)
l = Line()
l.add_tip(tip_length = 1, tip_width = 2)
a1.shift(UP)
l.shift(DOWN)
self.play(Write(a1), Write(l))
self.wait()
Output:
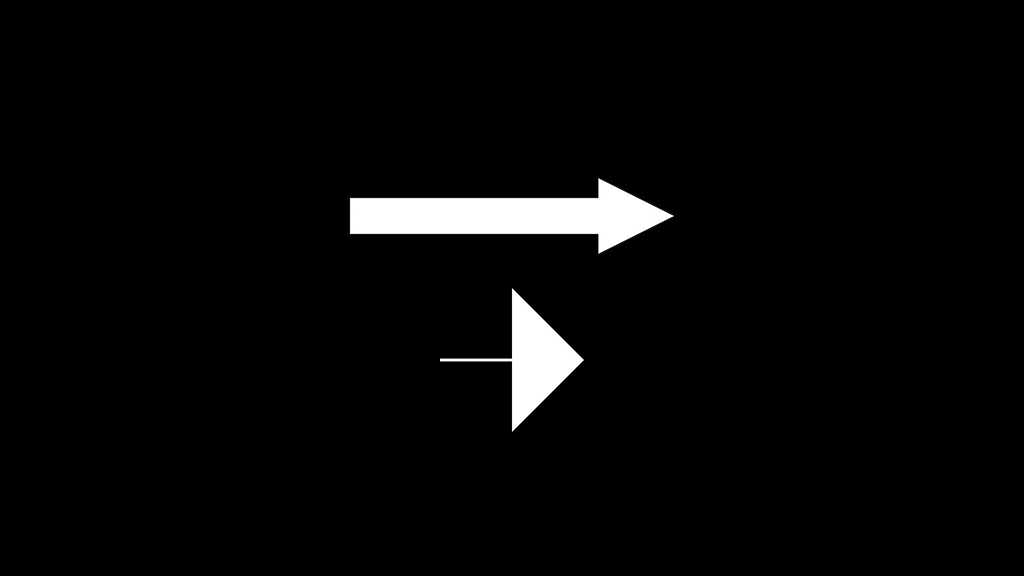
Code explanation:
Line 1: I imported the Manim library into Python.
Lines 3 and 4: I created a new Manim scene and named it “arrows”
Line 5: I created the first arrow and assigned it to a new variable named a1. I gave this arrow the stroke width of 50 but since the default maximum stroke width to length ratio wouldn’t allow the arrow to become this thicc I also increased the max_stroke_width_to_lenght_ratio.
Line 6: I scaled the first arrow by 3 times. By default, the tip of the arrow doesn’t scale with it so I also said scale_tips = True so that the whole arrow gets bigger not only the tail.
Line 8: I created a default line and assigned it to a variable I named l.
Line 9: I added an arrow tip to the line with the .add_tip() command and increased the tip’s length as well as width.
Line 11: I moved the first arrow up. You can learn more about how to position objects in Manim here.
Line 12: I moved the second arrow (or the line with an arrow tip) down.
Line 14: I animated both arrows on the screen using the self.play() command and the Write() animation type for each of them.
Line 15: I paused the animation for 1 second.
With this example, I wanted to show that you can adjust the tip size and the thickness of arrows in Manim differently depending on if you create that arrow with the Arrow() command or the line() command.
How to point an arrow to an object
To point an arrow to an object in Manim you have to define the end parameter.
Example:
from manim import *
class point_arrow(Scene):
def construct(self):
r = Rectangle(width = 0.5, height = 0.5, color = RED, fill_opacity = 1)
r.move_to([5, 3, 0])
a = Arrow(start = (3, -1, 0), end = r.get_bottom())
self.play(Write(r), Write(a))
self.wait()
Output:
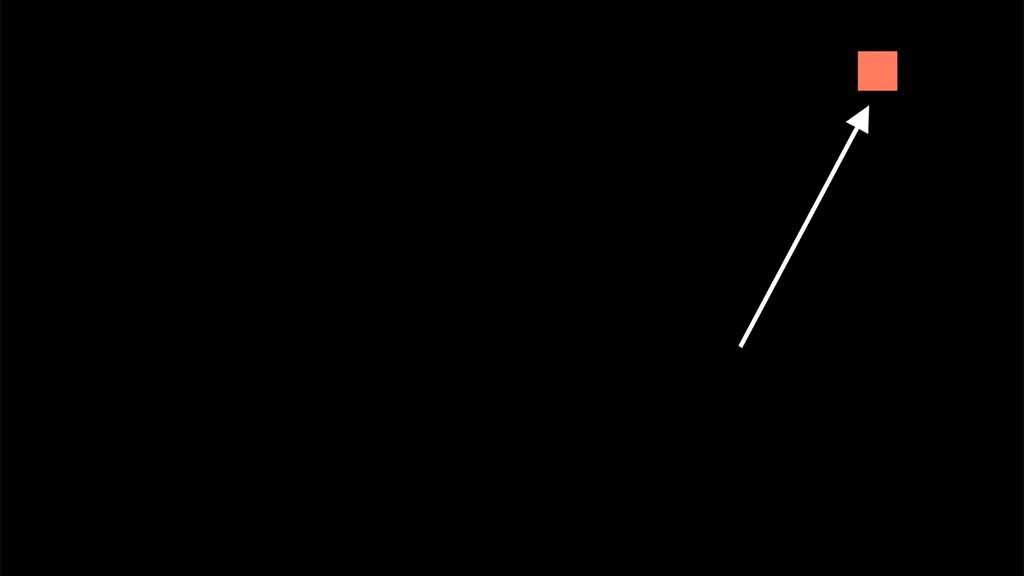
Code explanation:
Line 1: I imported the Manim library into Python.
Lines 3 and 4: I created a new Manim scene and named it “point_arrow”
Line 5: I created a red rectangle with a height and width of 0.5 and a fill opacity of 100%. I then assigned it to a new variable that I named r.
Line 6: I moved the rectangle to coordinates (x = 5; y = 3; z = 0) using the move_to() command. The z coordinate is always 0 when working in a 2D environment.
Line 7: I created an arrow and defined the start of it to begin at coordinates (x = 3, y = -1, z = 0) and the end of it to be at the bottom of the rectangle. I used the get_bottom() command to get the bottom of the rectangle. You can also do get_left(), get_right() and so on.
Line 9: I played the rectangle and the arrow on the screen using the Write() animation type for each.
Line 10: I paused the animation on the screen for 1 second.
How to create an arrow between two objects in Manim
To create an arrow between two objects you can use the DoubleArrow() command and then define the start and the end of the arrow.
Example:
from manim import *
class double_arrow(Scene):
def construct(self):
r = Rectangle(width = 0.5, height = 0.5, color = RED)
r.shift(RIGHT*3)
c = Circle(radius = 0.25, color = RED)
c.shift(LEFT*3)
a = DoubleArrow(start = c.get_right(), end = r.get_left(), tip_shape_start = StealthTip, tip_shape_end = StealthTip)
self.play(Write(r), Write(c), Write(a))
self.wait()
Output:
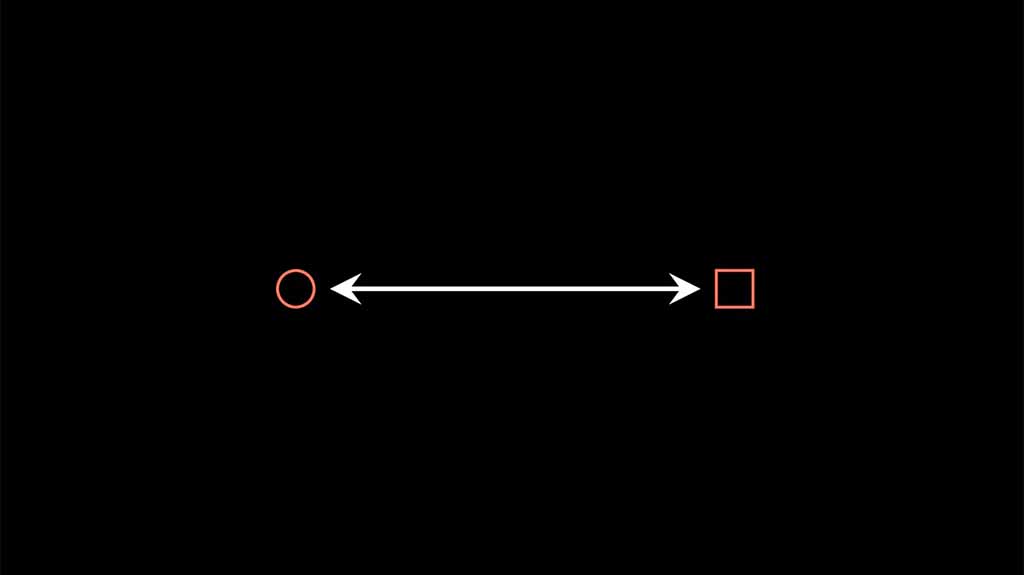
Code explanation:
Line 1: I imported the Manim library into Python.
Lines 3 and 4: I created a new Manim scene and named it “double_arrow”
Line 5: I created a red rectangle with a height and width of 0.5 and assigned it to a new variable I named r.
Line 6: I shifted the rectangle right by 3 squares in the coordinate system.
Line 8: I created a red circle with a radius of 0.25 so that it’s the same size as the rectangle. I assigned it to a new variable that I named c.
Line 9: I shifted the circle left by 3 squares using the .shift() command.
Line 11: I created a double arrow with the DoubleArrow() command and assigned it to a new variable a. I said that the arrow should start at the right side of the circle and end at the left side of the rectangle. I also changed both tips of the arrow to the StealthTip shape.
Line 14: I animated the rectangle, the circle, and the arrow on the screen using the Write() animation type for each.
Line 15: I paused the animation for 1 second.
How to animate an arrow moving with the object in Manim
To animate an arrow following an object in Manim you have to use an updater to redraw the arrow’s position on the screen as the object moves.
from manim import *
class redraw_arrow(Scene):
def construct(self):
c = Circle(0.25).shift(UP*3)
a = always_redraw(lambda: Arrow(end = c.get_center(), buff = 0.45))
self.play(Write(c), Write(a))
self.play(c.animate.shift(LEFT*3))
self.play(c.animate.shift(RIGHT*6))
self.play(c.animate.shift(DOWN*3))
self.wait()
self.play(Unwrite(c), Unwrite(a))
Output:
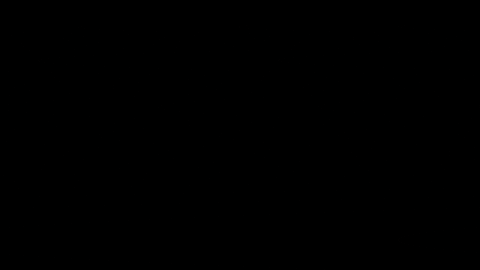
Code explanation:
Line 1: I imported Manim into Python.
Lines 3 and 4: I created a new Manim scene and named it “redraw_arrow”
Line 5: I created a circle with a radius of 0.25 and shifted it up by 3 squares in the coordinate system.
Line 6: I created an arrow and pointed it to the center of the circle by saying end = c.get_center(). I also gave the arrow a buff value of 0.45. The buff value defines the gap between two mobjects (in this case the arrow and the center of the circle). Finally, I added an always_redraw(lambda: ) updater to the arrow. Adding this to a mobject redraws it on the screen for every new frame of the animation. So in this case the arrow will always be redrawn to point to the center of the circle and always have a buff value of 0.45.
Line 8: I animated the arrow and the circle on the screen using the Write() animation type.
Line 10: I animated the circle moving left by 3 squares. To animate any object moving on the screen you have to attach the .animate attribute to it inside the self.play() command as well as define how you want to move it. In this case, I used the .shift() command to move the circle but I could have also used commands like move_to() or next_to().
Line 11: I animated the circle moving right by 6 squares.
Line 12: I animated the circle moving down by 3 squares.
Line 13: I removed the circle and the arrow from the screen by using the Unwrite() animation type.
In this example, anywhere that the object (circle) moves the arrow will try to point at it because it’s always redrawn to be pointing to the center of it with a buff value of 0.45.
Let me help you learn Manim
If you want to skip the headache of trying to learn Manim from a bunch of scattered information, I put together a comprehensive 3-hour Manim course for complete beginners.
It will give you all the foundational skills you need to start creating stunning animations with code.
Enroll In Manim Course For Beginners