There are 2 ways that you can play two or more animations in Manim at the same time.
One is by using a VGruop() to group objects together and then play that group on the screen; the second is by providing multiple animation commands inside the self.play() command.
Let’s explore both of them.
How to play multiple animations in Manim at the same time with multiple parameters in the self.play() command
The first way you can play multiple animations simultaneously in Manim is by providing multiple animation types inside of the same self.play() command separated with commas.
Example:
from manim import *
class play_at_the_same_time(Scene):
def construct(self):
s1 = Square(color = RED)
s2 = Square(color = ORANGE)
s1.next_to(s2, LEFT)
s3 = Square(color = YELLOW).next_to(s2, RIGHT)
self.play(Write(s1), Write(s2), Create(s3))
self.wait()
Output:
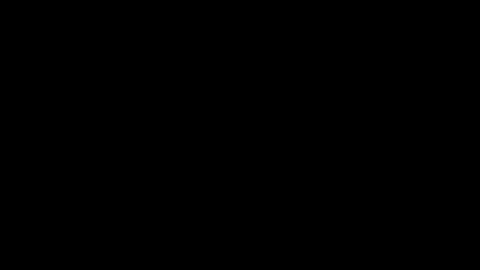
Code explanation by line:
Line 1: I imported the Manim library into VS Code so that it understands the code syntax.
Lines 3 and 4: I created a new Manim scene and named it “play_at_the_same_time”.
Lines 5: I created a red square and assigned it to a new variable that I named s1.
Line 6: I created an orange square and assigned it to a new variable that I named s2.
Line 7: I positioned the orange square (s2) next to the red square (s1) and on the left side of it. I used the next_to() command to do it. You can think of it like this: next_to(what, where). The reason I couldn’t attach this command to s1 directly (like I’m going to do with s3) was that I hadn’t yet created the s2 variable and Python interprets code from top to bottom. This means that if I had said s1 = Square(color = RED).next_to(s2, LEFT), Python wouldn’t have known what s2 is.
Line 8: I created a yellow square and assigned it to a new variable that I named s3. I then positioned this square next to the orange square using the next_to() command. To learn more about positioning in Manim I recommend checking out this guide: Manim: How To Align Objects.
Line 10: I animated all objects on the screen at the same time by putting all of their animations inside of the same self.play() command. Anytime you want to see something happening on the screen in Manim you have to use the self.play() command. I used the Write() animation type to animate the first two squares on the screen and the Create() animation type to animate the last square.
Line 10: I paused the animation for 1 second.
How to play multiple animations in Manim at the same time by using a VGroup()
The second way (and sometimes more convenient) is to group objects using a VGroup() and then play that whole group of objects on the screen at the same time.
Example:
from manim import *
class play_at_the_same_time2(Scene):
def construct(self):
s1 = Square(color = RED)
s2 = Square(color = ORANGE)
s3 = Square(color = YELLOW)
g = VGroup(s1, s2, s3)
g.arrange()
self.play(Write(g))
self.wait()
Output:
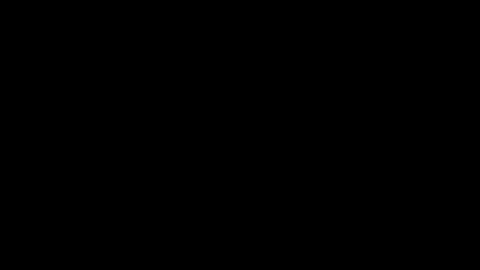
Code explanation:
Line 1: I imported the Manim library into Python.
Lines 3 and 4: I created a new Manim scene and named it “play_at_the_same_time2”.
Lines 5, 6, and 7: I created three new squares, red, orange, and yellow, and assigned each of them to new variables s1, s2, and s3.
Line 9: I grouped all of the squares using a VGroup(). When you group objects you can animate all of them at the same time.
Line 10: I arranged the VGroup() using the default arrangement method which arranges mobjects left to right and aligns them in the center of the screen. To learn more about what you can do with VGroups I recommend checking out this guide: Manim VGroup: Everything You Need To Know.
Line 12: I animated the group on the screen which allowed me to show all the objects inside of that group at the same time.
Line 13: I paused the animation for 1 second.
Let me help you learn Manim
If you want to skip the headache of trying to learn Manim from a bunch of scattered information, I put together a comprehensive 3-hour Manim course for complete beginners.
It will give you all the foundational skills you need to start creating stunning animations with code.
Enroll In Manim Course For Beginners